基于BP神经网络算法编程
时间: 2024-02-16 12:58:13 浏览: 167
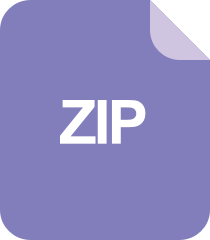
Python实现BP神经网络算法.zip
基于BP神经网络算法的编程可以通过Python来实现。下面是一个简单的例子来演示如何使用Python编写基于BP神经网络算法的程序:
```python
import numpy as np
# 定义激活函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义BP神经网络类
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 初始化权重
self.W1 = np.random.randn(self.input_size, self.hidden_size)
self.W2 = np.random.randn(self.hidden_size, self.output_size)
def forward(self, X):
# 前向传播
self.z = np.dot(X, self.W1)
self.z2 = sigmoid(self.z)
self.z3 = np.dot(self.z2, self.W2)
output = sigmoid(self.z3)
return output
def backward(self, X, y, output, learning_rate):
# 反向传播
self.output_error = y - output
self.output_delta = self.output_error * sigmoid(output, derivative=True)
self.z2_error = self.output_delta.dot(self.W2.T)
self.z2_delta = self.z2_error * sigmoid(self.z2, derivative=True)
self.W1 += X.T.dot(self.z2_delta) * learning_rate
self.W2 += self.z2.T.dot(self.output_delta) * learning_rate
def train(self, X, y, epochs, learning_rate):
for i in range(epochs):
output = self.forward(X)
self.backward(X, y, output, learning_rate)
def predict(self, X):
return self.forward(X)
# 创建训练数据
X = np.array([[0, 0, 1],
[0, 1, 1],
[1, 0, 1],
[1, 1, 1]])
y = np.array([[0],
[1],
[1],
[0]])
# 创建BP神经网络对象
nn = NeuralNetwork(input_size=3, hidden_size=4, output_size=1)
# 训练BP神经网络
nn.train(X, y, epochs=10000, learning_rate=0.1)
# 预测新数据
new_data = np.array([[1, 0, 0]])
prediction = nn.predict(new_data)
print("Prediction:", prediction)
```
这个例子演示了如何使用BP神经网络算法来进行二进制分类。首先,我们定义了一个激活函数sigmoid,然后创建了一个NeuralNetwork类来实现BP神经网络。在训练过程中,我们使用前向传播和反向传播算法来更新权重,最后使用训练好的神经网络进行预测。
阅读全文
相关推荐
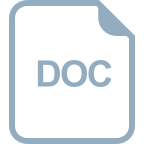
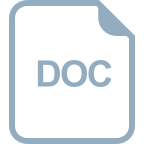
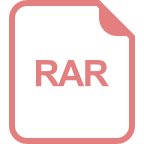
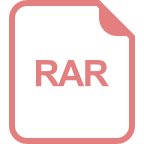
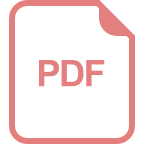
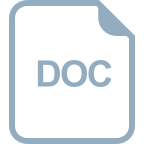

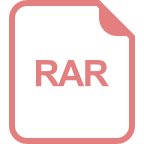
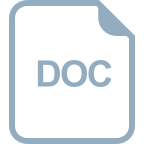
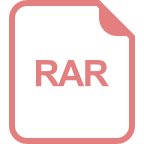
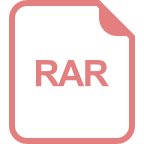
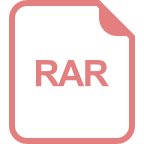
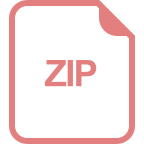
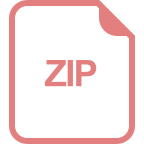
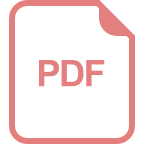
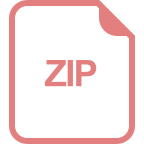
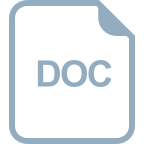
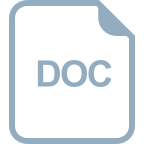