编写Java应用程序,执行此程序输出箱子的体积和重量。定义一个类,类中包含长、宽、高和比重,方法有:求体积和重量。构造方法定义时采用方法重载
时间: 2024-09-15 18:04:05 浏览: 94
在Java中,你可以编写一个名为Box的类,它将包含长(length)、宽(width)、高(height)属性以及比重(density)。这里我们将定义一个构造函数用于初始化长度、宽度和高度,并通过方法重载的方式分别计算体积和重量。以下是Box类的示例:
```java
public class Box {
private double length;
private double width;
private double height;
private double density; // 比重
// 构造方法,基础版本,所有维度都必须提供
public Box(double length, double width, double height, double density) {
this.length = length;
this.width = width;
this.height = height;
this.density = density;
}
// 构造方法,利用方法重载,允许只提供体积
public Box(double volume, double density) {
this.length = Math.cbrt(volume / (width * height)); // 假设长宽已知,仅基于体积计算
this.width = ... // 同理计算宽度
this.height = ... // 同理计算高度
this.density = density;
}
// 计算体积的方法,没有重载,因为只有一个
public double calculateVolume() {
return length * width * height;
}
// 计算重量的方法,采用重载,考虑体积和比重
public double calculateWeight() {
return calculateVolume() * density;
}
public static void main(String[] args) {
// 示例一:完整信息创建
Box box1 = new Box(10.0, 5.0, 3.0, 0.8); // 假设密度为0.8g/cm³
System.out.println("Box 1 Volume: " + box1.calculateVolume());
System.out.println("Box 1 Weight: " + box1.calculateWeight());
// 示例二:仅体积信息创建,自动计算其他尺寸
Box box2 = new Box(50.0, 0.8); // 假设体积为50cm³,密度不变
System.out.println("Box 2 Volume: " + box2.calculateVolume());
System.out.println("Box 2 Weight: " + box2.calculateWeight());
}
}
```
阅读全文
相关推荐
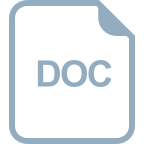
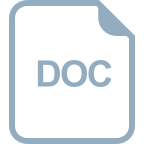
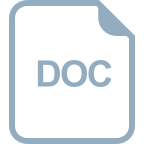
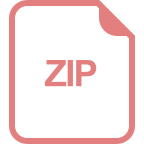
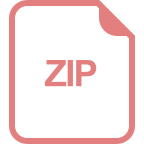
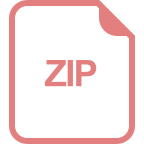
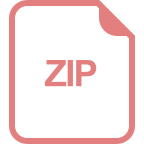
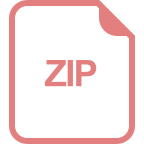
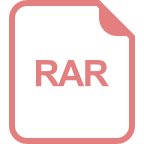
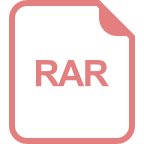
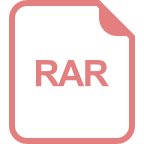
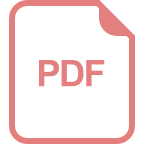
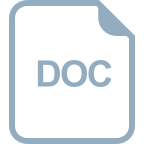
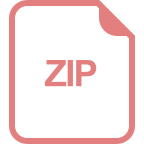
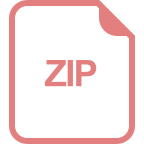
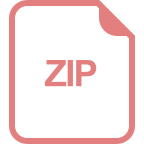
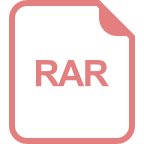
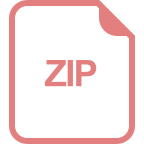