建立一个银行账号类,并提供存取操作要求1:deposit()时,如果金额<0,提示“Illegal transaction attempted” 要求2:withdraw()时,如果金额<0,提示“Illegal transaction attempted” 如果金额>余额,提示“Request denied due to insufficient funds”
时间: 2023-05-20 19:05:07 浏览: 121
好的,以下是建立一个银行账号类的代码:
class BankAccount:
def __init__(self, balance):
self.balance = balance
def deposit(self, amount):
if amount < 0:
print("Illegal transaction attempted")
else:
self.balance += amount
print("Deposit successful. New balance is:", self.balance)
def withdraw(self, amount):
if amount < 0:
print("Illegal transaction attempted")
elif amount > self.balance:
print("Request denied due to insufficient funds")
else:
self.balance -= amount
print("Withdrawal successful. New balance is:", self.balance)
请问还有什么问题吗?
相关问题
建立一个银行账号类,并提供存取操作
好的,以下是一个简单的银行账号类的代码示例:
```python
class BankAccount:
def __init__(self, account_number, balance=0):
self.account_number = account_number
self.balance = balance
def deposit(self, amount):
self.balance += amount
print(f"成功存入 {amount} 元,当前余额为 {self.balance} 元。")
def withdraw(self, amount):
if amount > self.balance:
print("余额不足,无法取款。")
else:
self.balance -= amount
print(f"成功取出 {amount} 元,当前余额为 {self.balance} 元。")
```
你可以使用这个类来创建银行账号对象,并进行存取操作。例如:
```python
my_account = BankAccount("1234567890")
my_account.deposit(1000) # 存入 1000 元
my_account.withdraw(500) # 取出 500 元
```
输出:
```
成功存入 1000 元,当前余额为 1000 元。
成功取出 500 元,当前余额为 500 元。
```
希望这个示例对你有所帮助!
用python:定义一个银行卡类,用于提供相关的开户、存款、取款、转帐、查询等操作
好的,根据您的要求,我为您提供一个Python实现的银行卡类:
```python
class BankAccount:
def __init__(self, account_no, balance=0):
self.account_no = account_no
self.balance = balance
def deposit(self, amount):
self.balance += amount
print(f"成功存入{amount}元,当前余额为{self.balance}元")
def withdraw(self, amount):
if self.balance < amount:
print("余额不足,取款失败")
else:
self.balance -= amount
print(f"成功取出{amount}元,当前余额为{self.balance}元")
def transfer(self, to_account, amount):
if self.balance < amount:
print("余额不足,转账失败")
else:
self.balance -= amount
to_account.deposit(amount)
print(f"成功转出{amount}元到账号{to_account.account_no},当前余额为{self.balance}元")
def query(self):
print(f"账号{self.account_no},当前余额为{self.balance}元")
```
使用方法:
```python
# 开户
account1 = BankAccount("123456")
account2 = BankAccount("654321")
# 存款
account1.deposit(1000)
# 成功存入1000元,当前余额为1000元
# 取款
account1.withdraw(500)
# 成功取出500元,当前余额为500元
# 转账
account1.transfer(account2, 300)
# 成功转出300元到账号654321,当前余额为200元
# 成功存入300元,当前余额为300元(账号654321)
# 查询余额
account1.query()
# 账号123456,当前余额为200元
```
希望我的回答能够解决您的问题!
相关推荐
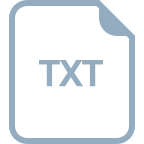
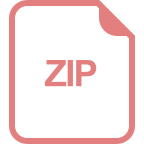
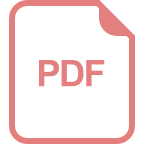












