c++设计活期存款类Deposit . 参考图1,每个账户有帐号(自动编号)、户名、密码、金额、月利率(静态成员)、开户时间6项信息。银行每月1号要根据账户余额发给客户利息,利息被累计到客户存款余额中。银行可以更改利率。 本月余额计算方法: 本月余额 = 上月余额 + 上月余额*月利率 建立账号,输出某一个日期后的账号信息 输入 第一行为开户账号的个数,后面为每行为账号信息,包括户名、密码、存款金额、月利率,开户时间 最后一行为结算日期 输出 输出结算后的账号信息(按
时间: 2023-06-19 07:03:33 浏览: 163
照账号自动编号升序排列),包含账号、户名、余额三项信息,余额保留两位小数。
参考代码如下:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
#include <iomanip>
using namespace std;
class Deposit {
private:
static double month_rate;
int account_id;
string name;
string password;
double balance;
string open_date;
public:
Deposit(string n, string p, double b, string d) {
static int id = 100000;
account_id = id++;
name = n;
password = p;
balance = b;
open_date = d;
}
int get_id() const { return account_id; }
string get_name() const { return name; }
double get_balance() const { return balance; }
string get_open_date() const { return open_date; }
static double get_month_rate() { return month_rate; }
static void set_month_rate(double rate) { month_rate = rate; }
void add_interest() { balance += balance * month_rate; }
};
double Deposit::month_rate = 0.01;
bool cmp(const Deposit& a, const Deposit& b) {
return a.get_id() < b.get_id();
}
int main() {
int n;
cin >> n;
vector<Deposit> accounts;
for (int i = 0; i < n; i++) {
string name, password, open_date;
double balance, month_rate;
cin >> name >> password >> balance >> month_rate >> open_date;
Deposit account(name, password, balance, open_date);
accounts.push_back(account);
}
string settle_date;
cin >> settle_date;
// 计算利息
for (auto& account : accounts) {
if (account.get_open_date() <= settle_date) {
int months = stoi(settle_date.substr(5, 2)) - stoi(account.get_open_date().substr(5, 2));
for (int i = 0; i < months; i++) {
account.add_interest();
}
}
}
// 按账号升序排序
sort(accounts.begin(), accounts.end(), cmp);
// 输出结果
for (const auto& account : accounts) {
if (account.get_open_date() <= settle_date) {
cout << account.get_id() << " " << account.get_name() << " " << fixed << setprecision(2) << account.get_balance() << endl;
}
}
return 0;
}
```
相关推荐
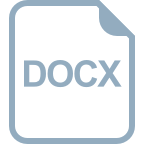
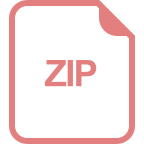















