c++设计活期存款类Deposit . 参考图1,每个账户有帐号(自动编号)、户名、密码、金额、月利率(静态成员)、开户时间6项信息。银行每月1号要根据账户余额发给客户利息,利息被累计到客户存款余额中。银行可以更改利率。
时间: 2023-12-16 09:04:00 浏览: 105
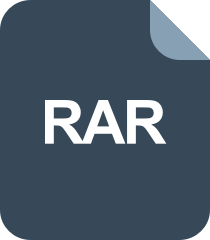
C++制作银行活期存款项目

好的,下面是 Deposit 类的设计:
```c++
#include <string>
#include <ctime>
class Deposit {
public:
Deposit(std::string name, std::string password, double money);
void deposit(double money);
void withdraw(double money);
void setInterestRate(double rate);
void addInterest();
void printInfo();
private:
std::string accountNumber;
std::string accountName;
std::string accountPassword;
double accountMoney;
static double interestRate; // 静态成员变量,月利率
time_t createTime; // 开户时间
};
double Deposit::interestRate = 0.01; // 初始利率为 1%
Deposit::Deposit(std::string name, std::string password, double money) {
// 自动生成账号
static int accountCount = 0;
accountNumber = "A" + std::to_string(accountCount++);
accountName = name;
accountPassword = password;
accountMoney = money;
createTime = time(nullptr); // 获取当前时间
}
void Deposit::deposit(double money) {
accountMoney += money;
}
void Deposit::withdraw(double money) {
if (money > accountMoney) {
std::cout << "余额不足!" << std::endl;
return;
}
accountMoney -= money;
}
void Deposit::setInterestRate(double rate) {
interestRate = rate;
}
void Deposit::addInterest() {
accountMoney += accountMoney * interestRate;
}
void Deposit::printInfo() {
std::cout << "账号:" << accountNumber << std::endl;
std::cout << "户名:" << accountName << std::endl;
std::cout << "密码:" << accountPassword << std::endl;
std::cout << "余额:" << accountMoney << std::endl;
std::cout << "月利率:" << interestRate << std::endl;
std::cout << "开户时间:" << ctime(&createTime) << std::endl;
}
```
这里通过构造函数自动生成账号,并且在添加或取出钱时进行余额判断。设置利率和添加利息的方法也都已经实现。
使用示例:
```c++
int main() {
Deposit d("Tom", "password", 1000.0); // 创建账户
d.printInfo();
std::cout << "存入 1000 元" << std::endl;
d.deposit(1000.0);
d.printInfo();
std::cout << "取出 500 元" << std::endl;
d.withdraw(500.0);
d.printInfo();
std::cout << "设置利率为 2%" << std::endl;
d.setInterestRate(0.02);
std::cout << "每月添加利息" << std::endl;
d.addInterest();
d.printInfo();
}
```
阅读全文
相关推荐












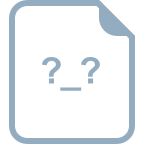
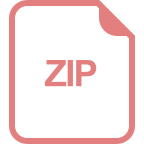
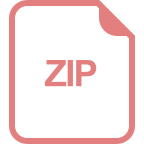
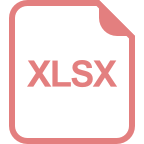