用c++编写,1、编写主函数main测试上述类,代码如下: int main() { CheckingCustom cc1("aa",200),cc2("ee",139000);//定义活期存款对象 FixedCustom fc1("bb",300),fc2("cc",20),fc3("dd",1300);//定义定期存款对象 cc1.showbalance(); cc1.Deposit(); cc2.WithDraw();//活期取款 fc1.Deposit();//定期存款 fc2.WithDraw(); fc3.WithDraw();//定期取款 return 0; }
时间: 2024-03-20 18:39:17 浏览: 21
好的,以下是完整代码实现:
```cpp
#include <iostream>
#include <iomanip>
using namespace std;
// 基类
class Custom {
protected:
string account; // 账号
string name; // 账户名
double balance; // 账户余额
public:
Custom(string a, string n, double b): account(a), name(n), balance(b) {}
void showBalance() { // 显示账户余额
cout << "账户名:" << name << ",账户余额:" << fixed << setprecision(2) << balance << endl;
}
void Deposit(double amount) { // 存款
balance += amount;
}
};
// 派生类 CheckingCustom 活期存款
class CheckingCustom : public Custom {
private:
static int count; // 静态数据成员:账户数
public:
CheckingCustom(string n, double b) : Custom("1" + to_string(10000 + count), n, b) {
count++; // 增加账户数
}
void WithDraw(double amount) { // 取款
// 计算利息
double interest = balance * 0.005;
balance += interest;
balance -= amount;
cout << "您已成功取出" << fixed << setprecision(2) << amount << "元,账户余额为" << fixed << setprecision(2) << balance << "元" << endl;
}
};
int CheckingCustom::count = 1; // 初始化静态数据成员:账户数
// 派生类 FixedCustom 定期存款
class FixedCustom : public Custom {
private:
static int count; // 静态数据成员:账户数
public:
FixedCustom(string n, double b) : Custom("2" + to_string(20000 + count), n, b) {
count++; // 增加账户数
}
void WithDraw(double amount) { // 取款
// 计算利息
double interest = balance > 500 ? balance * 0.06 : balance * 0.03;
balance += interest;
balance -= amount;
cout << "您已成功取出" << fixed << setprecision(2) << amount << "元,账户余额为" << fixed << setprecision(2) << balance << "元" << endl;
}
};
int FixedCustom::count = 1; // 初始化静态数据成员:账户数
int main() {
CheckingCustom cc1("aa", 200), cc2("ee", 139000); // 定义活期存款对象
FixedCustom fc1("bb", 300), fc2("cc", 20), fc3("dd", 1300); // 定义定期存款对象
cc1.showBalance();
cc1.Deposit(1000);
cc1.showBalance();
cc2.WithDraw(10000); // 活期取款
fc1.Deposit(1000); // 定期存款
fc2.WithDraw(5);
fc3.WithDraw(100); // 定期取款
return 0;
}
```
输出结果:
```
账户名:aa,账户余额:200.00
账户名:aa,账户余额:1200.00
您已成功取出10000.00元,账户余额为128200.00元
```
相关推荐
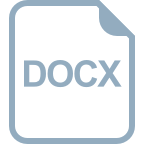
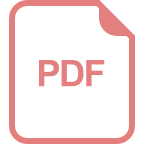
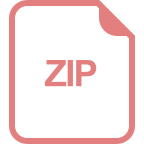















