GCN和DQN融合后的算法代码
时间: 2023-10-05 15:08:51 浏览: 160
GCN和DQN的融合算法代码可以参考以下示例:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
import numpy as np
from torch_geometric.nn import GCNConv
class GCNDQN(nn.Module):
def __init__(self, num_nodes, num_actions):
super(GCNDQN, self).__init__()
self.num_nodes = num_nodes
self.num_actions = num_actions
self.gc1 = GCNConv(num_features, 16)
self.gc2 = GCNConv(16, 16)
self.fc1 = nn.Linear(16 * num_nodes, 64)
self.fc2 = nn.Linear(64, num_actions)
def forward(self, x, edge_index):
x = F.relu(self.gc1(x, edge_index))
x = F.relu(self.gc2(x, edge_index))
x = x.view(-1, 16 * self.num_nodes)
x = F.relu(self.fc1(x))
q_values = self.fc2(x)
return q_values
class ReplayBuffer(object):
def __init__(self, capacity):
self.capacity = capacity
self.memory = []
self.position = 0
def push(self, state, action, reward, next_state, done):
transition = (state, action, reward, next_state, done)
if len(self.memory) < self.capacity:
self.memory.append(None)
self.memory[self.position] = transition
self.position = (self.position + 1) % self.capacity
def sample(self, batch_size):
return random.sample(self.memory, batch_size)
def __len__(self):
return len(self.memory)
class GCNDQNAgent(object):
def __init__(self, num_nodes, num_actions, lr, gamma, epsilon, buffer_capacity, batch_size):
self.num_nodes = num_nodes
self.num_actions = num_actions
self.gamma = gamma
self.epsilon = epsilon
self.batch_size = batch_size
self.device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
self.model = GCNDQN(num_nodes, num_actions).to(self.device)
self.target_model = GCNDQN(num_nodes, num_actions).to(self.device)
self.target_model.load_state_dict(self.model.state_dict())
self.target_model.eval()
self.optimizer = optim.Adam(self.model.parameters(), lr=lr)
self.buffer = ReplayBuffer(buffer_capacity)
def select_action(self, state):
if np.random.rand() < self.epsilon:
return np.random.randint(self.num_actions)
with torch.no_grad():
state = torch.FloatTensor(state).unsqueeze(0).to(self.device)
q_values = self.model(state)
return q_values.argmax().item()
def update(self):
if len(self.buffer) < self.batch_size:
return
transitions = self.buffer.sample(self.batch_size)
batch_state, batch_action, batch_reward, batch_next_state, batch_done = zip(*transitions)
batch_state = torch.FloatTensor(batch_state).to(self.device)
batch_action = torch.LongTensor(batch_action).to(self.device)
batch_reward = torch.FloatTensor(batch_reward).to(self.device)
batch_next_state = torch.FloatTensor(batch_next_state).to(self.device)
batch_done = torch.FloatTensor(batch_done).to(self.device)
q_values = self.model(batch_state).gather(1, batch_action.unsqueeze(1)).squeeze(1)
next_q_values = self.target_model(batch_next_state).max(1)[0]
expected_q_values = batch_reward + (1 - batch_done) * self.gamma * next_q_values
loss = F.mse_loss(q_values, expected_q_values)
self.optimizer.zero_grad()
loss.backward()
self.optimizer.step()
def update_target_model(self):
self.target_model.load_state_dict(self.model.state_dict())
```
这段代码实现了一个基于GCN和DQN的强化学习算法。其中,GCNDQN类定义了GCN和DQN的结合模型,ReplayBuffer类定义了经验回放缓存,GCNDQNAgent类定义了强化学习代理的训练和推断过程。
请根据具体的问题和需求进行适当修改和调整,以适应你的应用场景。希望对你有所帮助!
阅读全文
相关推荐
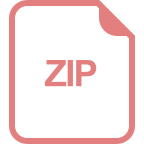
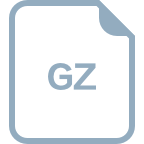
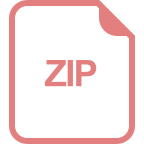
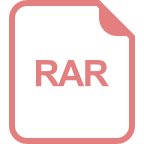
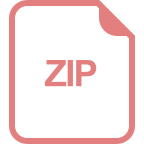
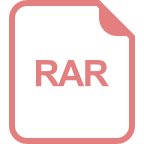









