将mysql连接到一个UI界面中,并实现自动存储数据和查找功能
时间: 2023-07-15 14:15:34 浏览: 120
要将MySQL连接到UI界面中,您需要使用一种编程语言(如Python)和相应的库(如PyQt或Tkinter),以便在UI中创建数据库连接并执行查询和插入操作。
下面是一个简单的例子,使用Python和PyQt库将MySQL连接到UI中,并实现自动存储数据和查找功能:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QLabel, QLineEdit, QPushButton
import mysql.connector
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建UI元素
self.centralWidget = QWidget(self)
self.setCentralWidget(self.centralWidget)
self.label1 = QLabel('Name:', self.centralWidget)
self.line1 = QLineEdit(self.centralWidget)
self.label2 = QLabel('Age:', self.centralWidget)
self.line2 = QLineEdit(self.centralWidget)
self.button1 = QPushButton('Save', self.centralWidget)
self.label3 = QLabel('Search:', self.centralWidget)
self.line3 = QLineEdit(self.centralWidget)
self.button2 = QPushButton('Find', self.centralWidget)
# 设置UI元素的位置和大小
self.label1.setGeometry(50, 50, 50, 30)
self.line1.setGeometry(100, 50, 150, 30)
self.label2.setGeometry(50, 100, 50, 30)
self.line2.setGeometry(100, 100, 150, 30)
self.button1.setGeometry(100, 150, 100, 30)
self.label3.setGeometry(50, 200, 50, 30)
self.line3.setGeometry(100, 200, 150, 30)
self.button2.setGeometry(100, 250, 100, 30)
# 连接MySQL数据库
self.conn = mysql.connector.connect(user='yourusername', password='yourpassword',
host='localhost', database='yourdatabase')
self.cursor = self.conn.cursor()
# 将保存按钮绑定到插入数据的函数
self.button1.clicked.connect(self.insertData)
# 将查找按钮绑定到查找数据的函数
self.button2.clicked.connect(self.findData)
def insertData(self):
# 从文本框中获取数据
name = self.line1.text()
age = int(self.line2.text())
# 将数据插入到MySQL数据库中
query = "INSERT INTO people (name, age) VALUES (%s, %s)"
values = (name, age)
self.cursor.execute(query, values)
self.conn.commit()
def findData(self):
# 从文本框中获取要查找的名字
name = self.line3.text()
# 查找MySQL数据库中符合条件的数据
query = "SELECT * FROM people WHERE name LIKE %s"
value = ('%' + name + '%', )
self.cursor.execute(query, value)
result = self.cursor.fetchall()
# 在控制台中打印结果
for row in result:
print(row)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
请注意,上面的示例仅用于演示如何将MySQL连接到UI中。在实际应用中,您需要添加更多功能和安全性措施,例如数据验证和防止SQL注入攻击。
相关推荐
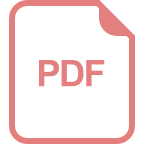
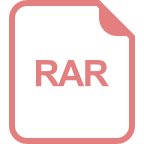
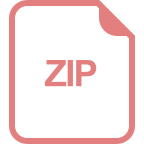














