生成一个用python爬取网页视频的代码
时间: 2024-03-24 09:35:14 浏览: 92
当然,以下是一个用Python爬取网页视频的示例代码:
```python
import requests
def download_video(url, save_path):
response = requests.get(url, stream=True)
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
file.write(chunk)
# 示例使用的视频链接
video_url = 'https://example.com/video.mp4'
# 视频保存路径
save_path = 'path/to/save/video.mp4'
download_video(video_url, save_path)
```
这段代码使用了`requests`库来发送HTTP请求,并通过流式写入的方式将视频数据保存到本地文件中。你只需要将`video_url`替换为你要爬取的视频链接,将`save_path`替换为你想要保存视频的路径即可。
相关问题
生成一个Python爬取图片的代码
可以这样写:import requests
from bs4 import BeautifulSoupurl = "https://example.com/images"# 获取页面内容
response = requests.get(url)
data = response.text# 使用BeautifulSoup解析页面
soup = BeautifulSoup(data, 'lxml')# 找到所有img标签
img_tags = soup.find_all('img')# 找到图片的URL
urls = [img['src'] for img in img_tags]# 下载图片
for url in urls:
response = requests.get(url)
file_name = url.split('/')[-1]
with open(file_name, 'wb') as f:
f.write(response.content)
生成一段python爬取网页脚本
好的,以下是一个简单的 Python 爬取网页脚本示例:
```python
import requests
url = "https://www.example.com"
response = requests.get(url)
if response.status_code == 200:
# 如果请求成功,输出网页源代码
print(response.text)
else:
# 如果请求失败,输出错误信息
print("请求失败,状态码为:", response.status_code)
```
这段代码使用了 Python 的 requests 库,发送 GET 请求获取指定网页的源代码,并根据返回的状态码进行处理。您可以根据自己的需求自定义请求的 URL 和处理方式。
阅读全文
相关推荐
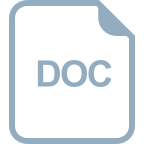
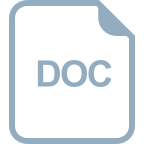
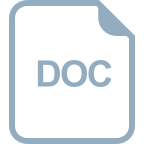
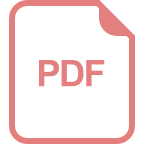
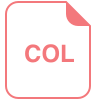






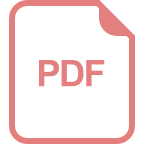



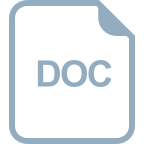
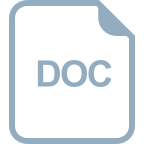