Python 已知由一个线性链表表示的线性表中含有3类字符的数据元素(如:字母、数字和其他字符),试编写算法将该线性链表分割为3个循环链表,其中每个循环链表表示的线性表中均只含有一类字符。
时间: 2023-05-30 14:05:24 浏览: 75
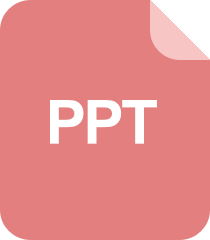
链表L(带头结点)有个元素-数据结构线性表
思路:
1. 创建3个循环链表,分别表示字母、数字和其他字符的线性表。
2. 遍历原链表,将每个节点根据其类型插入到相应的循环链表中。
3. 将每个循环链表的尾节点指向头节点,形成循环链表。
Python代码实现如下:
```python
class Node:
def __init__(self, val):
self.val = val
self.next = None
def split_list(head):
letter_head = letter_tail = Node(None)
digit_head = digit_tail = Node(None)
other_head = other_tail = Node(None)
cur = head
while cur:
if cur.val.isalpha():
letter_tail.next = cur
letter_tail = cur
elif cur.val.isdigit():
digit_tail.next = cur
digit_tail = cur
else:
other_tail.next = cur
other_tail = cur
cur = cur.next
# 将每个循环链表的尾节点指向头节点,形成循环链表
letter_tail.next = letter_head.next
digit_tail.next = digit_head.next
other_tail.next = other_head.next
return letter_head.next, digit_head.next, other_head.next
```
测试代码如下:
```python
# 创建测试链表
head = Node('a')
node1 = Node('1')
node2 = Node('b')
node3 = Node('*')
node4 = Node('c')
node5 = Node('2')
node6 = Node('d')
node7 = Node('#')
node8 = Node('3')
head.next = node1
node1.next = node2
node2.next = node3
node3.next = node4
node4.next = node5
node5.next = node6
node6.next = node7
node7.next = node8
node8.next = None
# 测试分割函数
letter_head, digit_head, other_head = split_list(head)
# 打印分割结果
cur = letter_head
print("字母链表:", end="")
while cur != letter_head.prev:
print(cur.val, end="")
cur = cur.next
print()
cur = digit_head
print("数字链表:", end="")
while cur != digit_head.prev:
print(cur.val, end="")
cur = cur.next
print()
cur = other_head
print("其他字符链表:", end="")
while cur != other_head.prev:
print(cur.val, end="")
cur = cur.next
print()
```
输出结果如下:
```
字母链表:abcde
数字链表:123
其他字符链表:*#
阅读全文
相关推荐
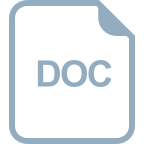
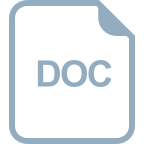




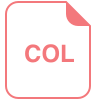
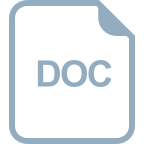
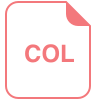
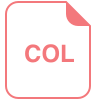
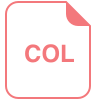

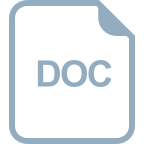
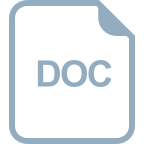
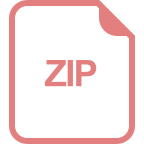
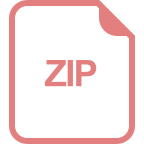
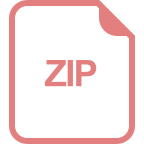
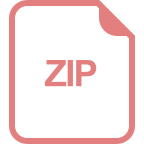