用c语言编写一个函数,实现在顺序存储方式下字符串的replace(S,T1,T2)运算。
时间: 2024-12-01 15:29:11 浏览: 12
在C语言中,你可以创建一个名为`replace_string`的函数,该函数接受三个参数:源字符串`s`, 需要查找并替换的子串`t1`以及替换的新子串`t2`。下面是一个简单的实现,使用了两个嵌套循环,第一个循环遍历源字符串,第二个循环用于替换找到的子串:
```c
#include <stdio.h>
#include <string.h>
void replace_string(char *s, char *t1, char *t2) {
int i, j, k;
int t1_len = strlen(t1);
// 检查t1是否为空
if (t1_len == 0) {
printf("Error: Target substring cannot be empty.\n");
return;
}
for (i = 0; s && s[i]; i++) { // 遍历整个源字符串
if (strncmp(&s[i], t1, t1_len) == 0) { // 找到t1的起始位置
// 使用指针计算替换范围
j = i;
while (j < i + t1_len && s[j]) {
j++;
}
// 用新子串替换旧子串
k = 0;
while (k < t1_len && s[j-k]) {
s[i+k] = t2[k];
k++;
}
i = j - 1; // 移动索引到新子串结束的位置
}
}
}
// 测试函数
int main() {
char str[] = "hello world";
char t1[] = "world";
char t2[] = "universe";
replace_string(str, t1, t2);
printf("Replaced string: %s\n", str);
return 0;
}
```
注意:此代码会直接修改输入的`str`,如果你不想改变原始字符串,可以在调用`replace_string`之前复制一份。
阅读全文
相关推荐
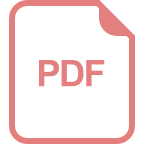
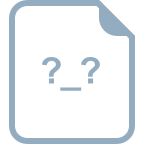









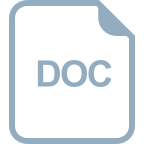
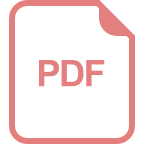
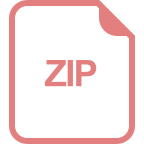
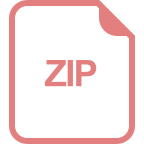