Java对象文件sleep线程动态演示
时间: 2024-03-05 07:52:00 浏览: 54
如果您想动态演示Java对象文件加密过程,可以使用Java Swing提供的GUI组件。以下是一个简单的例子:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
public class FileEncryptorGUI extends JFrame {
private final JTextField inputField;
private final JTextField outputField;
private final JPasswordField passwordField;
private final JButton encryptButton;
private final JTextArea logArea;
public FileEncryptorGUI() {
super("File Encryptor");
// Initialize components
inputField = new JTextField(20);
outputField = new JTextField(20);
passwordField = new JPasswordField(20);
encryptButton = new JButton("Encrypt");
logArea = new JTextArea(10, 30);
// Add components to content pane
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(createInputPanel(), BorderLayout.NORTH);
contentPane.add(new JScrollPane(logArea), BorderLayout.CENTER);
// Set window properties
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
private JPanel createInputPanel() {
JPanel inputPanel = new JPanel(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.anchor = GridBagConstraints.WEST;
gbc.insets = new Insets(5, 5, 5, 5);
inputPanel.add(new JLabel("Input file:"), gbc);
gbc.gridx = 1;
gbc.fill = GridBagConstraints.HORIZONTAL;
inputPanel.add(inputField, gbc);
gbc.gridx = 0;
gbc.gridy = 1;
gbc.fill = GridBagConstraints.NONE;
inputPanel.add(new JLabel("Output file:"), gbc);
gbc.gridx = 1;
gbc.fill = GridBagConstraints.HORIZONTAL;
inputPanel.add(outputField, gbc);
gbc.gridx = 0;
gbc.gridy = 2;
gbc.fill = GridBagConstraints.NONE;
inputPanel.add(new JLabel("Password:"), gbc);
gbc.gridx = 1;
gbc.fill = GridBagConstraints.HORIZONTAL;
inputPanel.add(passwordField, gbc);
gbc.gridx = 0;
gbc.gridy = 3;
gbc.gridwidth = 2;
gbc.fill = GridBagConstraints.NONE;
gbc.anchor = GridBagConstraints.CENTER;
inputPanel.add(encryptButton, gbc);
// Add action listener to encrypt button
encryptButton.addActionListener(this::encryptFile);
return inputPanel;
}
private void encryptFile(ActionEvent event) {
// Disable encrypt button
encryptButton.setEnabled(false);
// Clear log area
logArea.setText("");
// Get input file path, output file path, and password from input fields
String inputFilePath = inputField.getText();
String outputFilePath = outputField.getText();
String password = new String(passwordField.getPassword());
// Perform file encryption in separate thread
Thread encryptThread = new Thread(() -> {
// Read input file
List<Byte> inputFileBytes = new ArrayList<>();
try (FileInputStream inputStream = new FileInputStream(inputFilePath)) {
int byteValue;
while ((byteValue = inputStream.read()) != -1) {
inputFileBytes.add((byte) byteValue);
}
} catch (IOException e) {
logError("Error reading input file: " + e.getMessage());
return;
}
// Encrypt file
List<Byte> outputFileBytes = new ArrayList<>();
for (int i = 0; i < inputFileBytes.size(); i++) {
byte inputByte = inputFileBytes.get(i);
byte passwordByte = (byte) password.charAt(i % password.length());
byte outputByte = (byte) (inputByte ^ passwordByte);
outputFileBytes.add(outputByte);
logMessage(String.format("Encrypted byte %d/%d", i + 1, inputFileBytes.size()));
// Sleep for 1 second
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
logError("Encryption interrupted: " + e.getMessage());
return;
}
}
// Write output file
try (FileOutputStream outputStream = new FileOutputStream(outputFilePath)) {
for (Byte outputByte : outputFileBytes) {
outputStream.write(outputByte);
}
logMessage("Encryption complete!");
} catch (IOException e) {
logError("Error writing output file: " + e.getMessage());
return;
}
// Re-enable encrypt button
encryptButton.setEnabled(true);
});
encryptThread.start();
}
private void logMessage(String message) {
logArea.append(message + "\n");
}
private void logError(String error) {
logMessage("Error: " + error);
}
public static void main(String[] args) {
new FileEncryptorGUI();
}
}
```
这个程序会创建一个GUI窗口,用户可以在窗口中输入要加密的文件路径、加密后文件的路径和密码。当用户点击“Encrypt”按钮时,程序会在新线程中对文件进行加密,并在GUI窗口中展示加密过程的日志信息,包括每个字节的加密进度和加密完成的消息。在加密过程中,程序会每隔1秒钟暂停一次,以模拟加密过程的延迟。
阅读全文
相关推荐
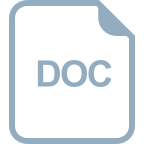
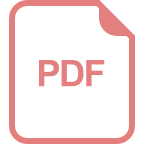
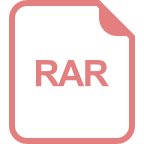
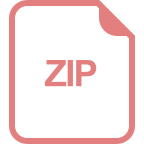
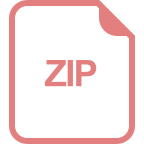
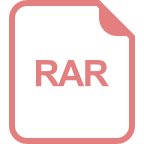
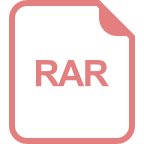
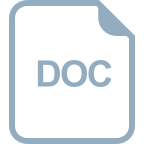
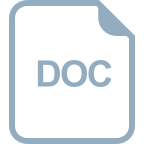
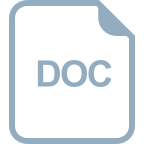
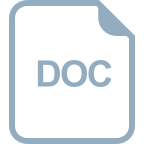
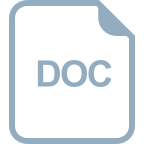
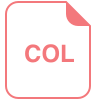
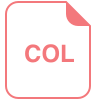
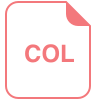
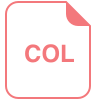


