在python中,os.rmdir函数如何强制删除
时间: 2023-05-28 22:03:27 浏览: 120
在Python中,os.rmdir函数默认情况下只能删除空文件夹,如果文件夹中有文件或者子文件夹,则会报错。如果要强制删除非空文件夹,可以使用os模块中的shutil.rmtree函数,该函数会递归删除文件夹及其子文件夹和文件。
示例代码:
```python
import shutil
# 删除非空文件夹
shutil.rmtree('path/to/folder', ignore_errors=True)
```
其中,`path/to/folder`是要删除的文件夹路径,`ignore_errors=True`表示忽略错误,即如果文件夹不存在或者无法删除,也不会报错。
相关问题
使用 Python 的 os.path 模块中的 exists() 函数来判断文件夹是否存在,存在就删除
可以通过结合使用 `os.path.exists()` 和 `os.rmdir()` 函数来判断文件夹是否存在并删除。下面是一个示例代码:
```python
import os
folder_path = '/path/to/folder'
if os.path.exists(folder_path):
os.rmdir(folder_path)
print(f'已删除文件夹 {folder_path}')
else:
print(f'文件夹 {folder_path} 不存在')
```
在上面的代码中,首先使用 `os.path.exists()` 函数判断给定路径的文件夹是否存在。如果存在,就使用 `os.rmdir()` 函数删除该文件夹。最后输出相应的结果。请确保在 `folder_path` 变量中替换为实际的文件夹路径。
帮我用python优化一下以下代码:def scanFile(filePath): # fp = open("folder.log", "w+") for path in os.walk(filePath): lists.append(path[0]) for i in lists: try: if len(os.listdir(i)) != 0: continue else: ls.append(i) except: continue for m in range(len(ls)): for x in ls: os.rmdir(ls[m]) with open("folder.log", "a+") as f: data = ls[m] f.write(data) f.write('\n') f.close() print(datetime.now(), "正在删除", ls[m]) ls.clear() scanFile(filePath) # fp.close()
你好,很高兴为你解答。
下面是一种使用 Python 优化 `scanFile` 函数的方法:
1. 使用 with 语句打开文件,这样可以保证在读取完文件后正确关闭文件。
例如:
```
def scanFile(filePath):
with open(filePath, 'r') as f:
# 读取文件内容
contents = f.read()
# 在这里处理文件内容
# ...
```
2. 将文件内容读取为列表,这样可以使用列表推导式(list comprehension)或 map 函数对列表中的每一行进行处理。
例如:
```
def scanFile(filePath):
with open(filePath, 'r') as f:
lines = f.readlines()
# 使用列表推导式对每一行进行处理
processed_lines = [processLine(line) for line in lines]
# 使用 map 函数对每一行进行处理
processed_lines = list(map(processLine, lines))
```
3. 将文件内容按行读取并处理,这样可以在处理完每一行后立即释放内存。
例如:
```
def scanFile(filePath):
with open(filePath, 'r') as f:
for line in f:
# 在这里处理每一行
processed_line = processLine(line)
# 在这里使用处理后的行
# ...
```
希望这些建议对你有帮助!
阅读全文
相关推荐
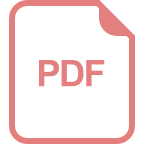
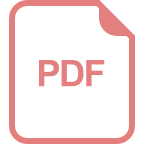
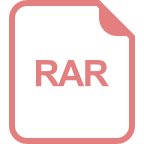
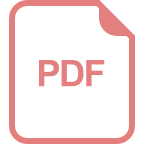
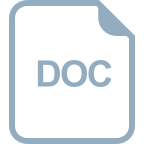
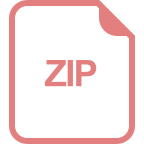
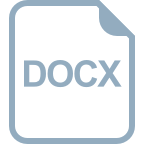
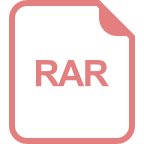
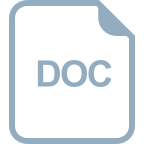
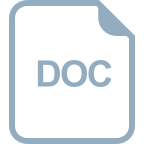
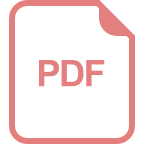
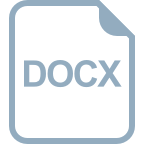
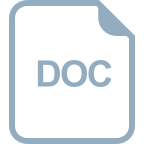
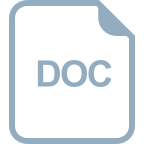



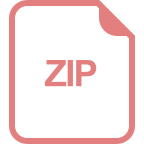