遗传算法优化切比雪夫神经网络代码
时间: 2023-09-03 12:12:05 浏览: 55
遗传算法可以用于优化神经网络的参数,以下是使用遗传算法优化切比雪夫神经网络的Python代码示例:
```python
import numpy as np
import random
# 定义网络结构
class ChebyshevNet:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 随机初始化权重
self.weights1 = np.random.randn(hidden_size, input_size)
self.weights2 = np.random.randn(output_size, hidden_size)
self.bias1 = np.random.randn(hidden_size)
self.bias2 = np.random.randn(output_size)
# 定义前向传播
def forward(self, x):
x = np.dot(self.weights1, x) + self.bias1
x = np.maximum(x, 0)
x = np.dot(self.weights2, x) + self.bias2
return x
# 定义适应度函数
def fitness(self, data, labels):
loss = 0
for i in range(data.shape[0]):
x = data[i]
y = labels[i]
y_pred = self.forward(x)
loss += np.max(np.abs(y_pred - y))
return -loss
# 定义遗传算法
class GeneticAlgorithm:
def __init__(self, population_size, mutation_rate, elite_rate, tournament_size):
self.population_size = population_size
self.mutation_rate = mutation_rate
self.elite_rate = elite_rate
self.tournament_size = tournament_size
# 生成随机种群
def generate_population(self, input_size, hidden_size, output_size):
population = []
for i in range(self.population_size):
net = ChebyshevNet(input_size, hidden_size, output_size)
population.append(net)
return population
# 计算每个个体的适应度
def evaluate_population(self, population, data, labels):
fitnesses = []
for net in population:
fitness = net.fitness(data, labels)
fitnesses.append(fitness)
return fitnesses
# 选择个体
def select_population(self, population, fitnesses):
selected = []
while len(selected) < self.population_size:
tournament = random.sample(range(len(population)), self.tournament_size)
tournament_fitnesses = [fitnesses[i] for i in tournament]
winner = tournament[np.argmax(tournament_fitnesses)]
selected.append(population[winner])
return selected
# 交叉个体
def crossover_population(self, population):
offspring = []
elite_size = int(self.elite_rate * self.population_size)
elite = population[:elite_size]
while len(offspring) < self.population_size - elite_size:
parent1, parent2 = random.sample(population, 2)
child1 = ChebyshevNet(parent1.input_size, parent1.hidden_size, parent1.output_size)
child2 = ChebyshevNet(parent1.input_size, parent1.hidden_size, parent1.output_size)
for i in range(parent1.hidden_size):
if random.random() < 0.5:
child1.weights1[i] = parent1.weights1[i]
child2.weights1[i] = parent2.weights1[i]
else:
child1.weights1[i] = parent2.weights1[i]
child2.weights1[i] = parent1.weights1[i]
for i in range(parent1.output_size):
if random.random() < 0.5:
child1.weights2[i] = parent1.weights2[i]
child2.weights2[i] = parent2.weights2[i]
else:
child1.weights2[i] = parent2.weights2[i]
child2.weights2[i] = parent1.weights2[i]
for i in range(parent1.hidden_size):
if random.random() < 0.5:
child1.bias1[i] = parent1.bias1[i]
child2.bias1[i] = parent2.bias1[i]
else:
child1.bias1[i] = parent2.bias1[i]
child2.bias1[i] = parent1.bias1[i]
for i in range(parent1.output_size):
if random.random() < 0.5:
child1.bias2[i] = parent1.bias2[i]
child2.bias2[i] = parent2.bias2[i]
else:
child1.bias2[i] = parent2.bias2[i]
child2.bias2[i] = parent1.bias2[i]
offspring.append(child1)
offspring.append(child2)
return elite + offspring
# 变异个体
def mutate_population(self, population):
for net in population:
for i in range(net.hidden_size):
if random.random() < self.mutation_rate:
net.weights1[i] += np.random.randn(net.input_size) * 0.1
for i in range(net.output_size):
if random.random() < self.mutation_rate:
net.weights2[i] += np.random.randn(net.hidden_size) * 0.1
for i in range(net.hidden_size):
if random.random() < self.mutation_rate:
net.bias1[i] += np.random.randn() * 0.1
for i in range(net.output_size):
if random.random() < self.mutation_rate:
net.bias2[i] += np.random.randn() * 0.1
return population
# 进化种群
def evolve_population(self, population, data, labels):
fitnesses = self.evaluate_population(population, data, labels)
population = self.select_population(population, fitnesses)
population = self.crossover_population(population)
population = self.mutate_population(population)
return population
# 定义数据集
data = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
labels = np.array([[0], [1], [1], [0]])
# 定义遗传算法参数
input_size = data.shape[1]
hidden_size = 10
output_size = labels.shape[1]
population_size = 100
mutation_rate = 0.1
elite_rate = 0.2
tournament_size = 5
# 生成随机种群
ga = GeneticAlgorithm(population_size, mutation_rate, elite_rate, tournament_size)
population = ga.generate_population(input_size, hidden_size, output_size)
# 进化种群
for i in range(100):
population = ga.evolve_population(population, data, labels)
# 选出最优个体
fitnesses = ga.evaluate_population(population, data, labels)
best_index = np.argmax(fitnesses)
best_net = population[best_index]
# 打印最优个体的预测结果
for i in range(data.shape[0]):
x = data[i]
y = labels[i]
y_pred = best_net.forward(x)
print("Input:", x, "Actual output:", y, "Predicted output:", y_pred)
```
在这个示例中,我们使用遗传算法优化一个切比雪夫神经网络来解决异或问题。我们定义了一个`ChebyshevNet`类来表示切比雪夫神经网络,它包含一个前向传播函数和一个适应度函数。我们还定义了一个`GeneticAlgorithm`类来实现遗传算法,它包含生成随机种群、计算适应度、选择、交叉和变异等操作。最后,我们使用遗传算法来进化种群并找到最优个体,然后使用最优个体来预测输入数据的输出。
相关推荐
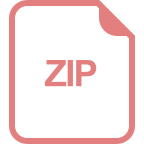
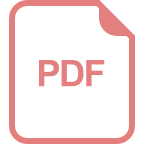














