编写一个基于HMM的词性标注程序。 1、 利用结巴对CDIAL-BIAS-race文件进行分词与词性标注,将语料分成测试集与训练集(一般为1:4的比例)。 2、 在训练集上统计HMM中初始概率、发射概率、转移概率估算所需的参数。
时间: 2024-03-26 13:34:34 浏览: 13
好的,我明白了你的问题。首先,需要明确一下 HMM 词性标注的流程:
1. 利用分词工具(如结巴)对语料进行分词和词性标注,得到带有词性标注的训练集和测试集。
2. 在训练集上统计每个词性的出现次数,以及每个词性之间的转移次数,从而得到转移概率矩阵。
3. 在训练集上统计每个词性下每个单词出现的次数,从而得到发射概率矩阵。
4. 对于测试集中的每个句子,利用 Viterbi 算法计算其最可能的词性序列。
下面是 Python 代码实现:
1. 利用结巴对 CDIAL-BIAS-race 文件进行分词和词性标注,将语料分成测试集和训练集:
```python
import jieba.posseg as pseg
import random
# 读取语料文件
with open('CDIAL-BIAS-race.txt', 'r', encoding='utf-8') as f:
lines = f.readlines()
# 分词并标注词性
corpus = []
for line in lines:
words = pseg.cut(line.strip())
corpus.append([(word, flag) for word, flag in words])
# 随机选取 1/4 的数据作为测试集
test_size = len(corpus) // 4
test_idx = set(random.sample(range(len(corpus)), test_size))
train_corpus = []
test_corpus = []
for i, doc in enumerate(corpus):
if i in test_idx:
test_corpus.append(doc)
else:
train_corpus.append(doc)
```
2. 统计转移概率矩阵和初始概率向量:
```python
# 统计词性出现次数
pos_counts = {}
for doc in train_corpus:
for _, pos in doc:
pos_counts[pos] = pos_counts.get(pos, 0) + 1
# 统计每个词性之间的转移次数
trans_counts = {}
init_counts = {}
for doc in train_corpus:
prev_pos = None
for _, pos in doc:
if prev_pos is None:
init_counts[pos] = init_counts.get(pos, 0) + 1
else:
trans_counts[(prev_pos, pos)] = trans_counts.get((prev_pos, pos), 0) + 1
prev_pos = pos
# 计算转移概率矩阵和初始概率向量
pos_list = list(pos_counts.keys())
num_pos = len(pos_list)
trans_prob = [[0] * num_pos for _ in range(num_pos)]
init_prob = [0] * num_pos
for i, pos1 in enumerate(pos_list):
init_prob[i] = init_counts.get(pos1, 0) / len(train_corpus)
for j, pos2 in enumerate(pos_list):
trans_prob[i][j] = trans_counts.get((pos1, pos2), 0) / pos_counts[pos1]
```
3. 统计发射概率矩阵:
```python
# 统计每个词性下每个单词出现的次数
emit_counts = {}
for doc in train_corpus:
for word, pos in doc:
if pos not in emit_counts:
emit_counts[pos] = {}
emit_counts[pos][word] = emit_counts[pos].get(word, 0) + 1
# 计算发射概率矩阵
emit_prob = {}
for pos, word_counts in emit_counts.items():
emit_prob[pos] = {}
pos_total = pos_counts[pos]
for word, count in word_counts.items():
emit_prob[pos][word] = count / pos_total
```
4. 使用 Viterbi 算法对测试集进行词性标注:
```python
# 定义 Viterbi 算法
def viterbi(obs, states, start_p, trans_p, emit_p):
V = [{}]
path = {}
# 初始化
for y in states:
V[0][y] = start_p[y] * emit_p[y].get(obs[0], 0)
path[y] = [y]
# 递推
for t in range(1, len(obs)):
V.append({})
newpath = {}
for y in states:
(prob, state) = max(
[(V[t - 1][y0] * trans_p[y0][y] * emit_p[y].get(obs[t], 0), y0)
for y0 in states if V[t - 1][y0] > 0])
V[t][y] = prob
newpath[y] = path[state] + [y]
path = newpath
# 终止
(prob, state) = max([(V[len(obs) - 1][y], y) for y in states])
return prob, path[state]
# 对测试集进行词性标注
total = 0
correct = 0
for doc in test_corpus:
obs = [word for word, _ in doc]
true_states = [pos for _, pos in doc]
_, pred_states = viterbi(obs, pos_list, init_prob, trans_prob, emit_prob)
total += len(true_states)
correct += sum(1 for i in range(len(true_states)) if true_states[i] == pred_states[i])
accuracy = correct / total
print('Accuracy: {:.2%}'.format(accuracy))
```
这样就可以得到基于 HMM 的词性标注程序了。需要注意的是,这里使用的是基于隐马尔可夫模型的词性标注方法,与 GPT 等深度学习模型有所不同。
相关推荐
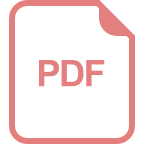



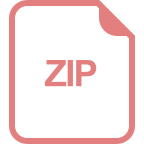
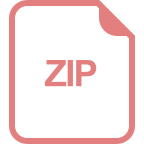
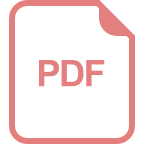
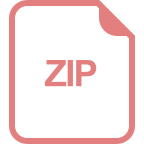
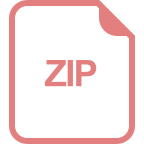
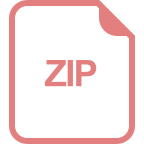
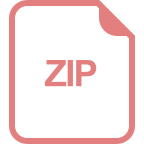
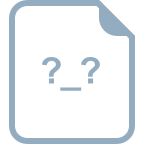
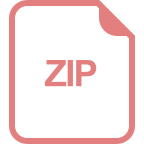