解释一下以下代码def start_game(): global score global color color = (randint(10, 255), randint(10, 255), randint(10, 255)) run_direction = "right" run = run_direction head = Position(160, 160) snake_body = [Position(head.x, head.y + 20), Position(head.x, head.y + 40), Position(head.x, head.y + 60)] food = Position(300, 300)
时间: 2024-04-02 20:34:21 浏览: 59
这段代码定义了一个名为 start_game 的函数,函数内部包含了一些变量的定义和初始化。
首先,函数中使用了两个全局变量 score 和 color,表示游戏分数和蛇的颜色。接着,使用 randint 函数生成一个随机的 RGB 颜色值,将其赋值给 color 变量。
接下来的代码定义了一些游戏运行时需要用到的变量,包括蛇的运动方向、蛇头的初始位置、蛇身体的初始位置、食物的初始位置等。其中,run_direction 变量表示蛇的运动方向,初始值为 "right",表示向右运动;head 变量表示蛇头的初始位置,初始值为 (160, 160);snake_body 变量表示蛇身体的初始位置,初始值为一个包含三个坐标点的列表,分别表示蛇头的下方20、40、60个像素处;food 变量表示食物的初始位置,初始值为 (300, 300)。
这段代码的作用是初始化游戏的一些变量,为后续的游戏运行做准备。
相关问题
帮我写出一段电脑游戏代码 就是单纯俄罗斯方块的小游戏代码
好的,下面是一个简单的俄罗斯方块游戏的代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 600
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置游戏标题
pygame.display.set_caption("俄罗斯方块")
# 定义方块的大小和颜色
BLOCK_SIZE = 20
BLOCK_COLOR = (0, 255, 0)
# 定义游戏区域的大小和位置
GAME_AREA_LEFT = 50
GAME_AREA_TOP = 50
GAME_AREA_WIDTH = 10 * BLOCK_SIZE
GAME_AREA_HEIGHT = 20 * BLOCK_SIZE
# 定义游戏区域的边框颜色和宽度
GAME_AREA_BORDER_COLOR = (255, 255, 255)
GAME_AREA_BORDER_WIDTH = 2
# 定义方块的形状
BLOCK_SHAPES = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的类型
BLOCK_TYPES = len(BLOCK_SHAPES)
# 定义方块的初始位置
INIT_BLOCK_X = 5
INIT_BLOCK_Y = 0
# 定义方块的移动速度
BLOCK_SPEED = 1
# 定义游戏的状态
GAME_STATE_START = 0
GAME_STATE_RUN = 1
GAME_STATE_OVER = 2
# 定义游戏得分
SCORE_PER_ROW = 100
# 定义游戏的字体
FONT_SIZE = 30
FONT_COLOR = (255, 255, 255)
font = pygame.font.SysFont(None, FONT_SIZE)
# 初始化游戏状态
game_state = GAME_STATE_START
# 初始化方块的位置和形状
block_x = INIT_BLOCK_X
block_y = INIT_BLOCK_Y
block_shape = BLOCK_SHAPES[random.randint(0, BLOCK_TYPES - 1)]
# 初始化游戏区域的矩阵
game_area = [[0] * 10 for i in range(20)]
# 初始化游戏得分
score = 0
# 绘制游戏区域的边框
def draw_game_area_border():
pygame.draw.rect(screen, GAME_AREA_BORDER_COLOR, (GAME_AREA_LEFT - GAME_AREA_BORDER_WIDTH, GAME_AREA_TOP - GAME_AREA_BORDER_WIDTH, GAME_AREA_WIDTH + 2 * GAME_AREA_BORDER_WIDTH, GAME_AREA_HEIGHT + 2 * GAME_AREA_BORDER_WIDTH), GAME_AREA_BORDER_WIDTH)
# 绘制方块
def draw_block():
for i in range(len(block_shape)):
for j in range(len(block_shape[i])):
if block_shape[i][j] != 0:
pygame.draw.rect(screen, BLOCK_COLOR, (GAME_AREA_LEFT + (block_x + j) * BLOCK_SIZE, GAME_AREA_TOP + (block_y + i) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制游戏区域
def draw_game_area():
for i in range(len(game_area)):
for j in range(len(game_area[i])):
if game_area[i][j] != 0:
pygame.draw.rect(screen, BLOCK_COLOR, (GAME_AREA_LEFT + j * BLOCK_SIZE, GAME_AREA_TOP + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 判断方块是否可以移动
def can_move(x, y, shape):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
if x + j < 0 or x + j >= 10 or y + i >= 20 or (y + i >= 0 and game_area[y + i][x + j] != 0):
return False
return True
# 移动方块
def move_block(x, y, shape):
if can_move(x, y, shape):
return (x, y, shape)
else:
return (block_x, block_y, block_shape)
# 固定方块
def fix_block(x, y, shape):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
game_area[y + i][x + j] = shape[i][j]
return (INIT_BLOCK_X, INIT_BLOCK_Y, BLOCK_SHAPES[random.randint(0, BLOCK_TYPES - 1)])
# 消除行
def remove_row():
global score
rows = []
for i in range(len(game_area)):
if sum(game_area[i]) == 10:
rows.append(i)
for row in rows:
del game_area[row]
game_area.insert(0, [0] * 10)
score += SCORE_PER_ROW * len(rows)
# 绘制游戏得分
def draw_score():
score_text = font.render("Score: " + str(score), True, FONT_COLOR)
screen.blit(score_text, (10, 10))
# 绘制游戏结束界面
def draw_game_over():
game_over_text = font.render("Game Over", True, FONT_COLOR)
screen.blit(game_over_text, (WINDOW_WIDTH // 2 - FONT_SIZE * 3, WINDOW_HEIGHT // 2 - FONT_SIZE))
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if game_state == GAME_STATE_START or game_state == GAME_STATE_OVER:
if event.key == pygame.K_SPACE:
game_state = GAME_STATE_RUN
block_x, block_y, block_shape = (INIT_BLOCK_X, INIT_BLOCK_Y, BLOCK_SHAPES[random.randint(0, BLOCK_TYPES - 1)])
game_area = [[0] * 10 for i in range(20)]
score = 0
elif game_state == GAME_STATE_RUN:
if event.key == pygame.K_LEFT:
block_x -= BLOCK_SPEED
elif event.key == pygame.K_RIGHT:
block_x += BLOCK_SPEED
elif event.key == pygame.K_DOWN:
block_y += BLOCK_SPEED
elif event.key == pygame.K_UP:
block_shape = [[block_shape[j][i] for j in range(len(block_shape))] for i in range(len(block_shape[0]))]
# 游戏逻辑
if game_state == GAME_STATE_RUN:
block_x, block_y, block_shape = move_block(block_x, block_y + BLOCK_SPEED, block_shape)
if block_y == INIT_BLOCK_Y and not can_move(block_x, block_y, block_shape):
game_state = GAME_STATE_OVER
else:
block_x, block_y, block_shape = fix_block(block_x, block_y, block_shape)
remove_row()
# 绘制游戏窗口
screen.fill((0, 0, 0))
draw_game_area_border()
draw_block()
draw_game_area()
draw_score()
if game_state == GAME_STATE_OVER:
draw_game_over()
pygame.display.update()
```
这段代码使用了 Pygame 库,运行时需要先安装 Pygame 库。
利用pygame模块实现飞机大战附加功能如下: (1) 实现碰撞检测(可以消灭敌机,也可以被敌机消灭) (2) 随机出现云朵在天空中漂浮 (3) 随机出现甜甜圈进行能量补给(生命力或子弹类型发生变化) (4) 有GAME OVER条件控制
好的,这里给出一份利用pygame模块实现飞机大战并添加上述附加功能的示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小和标题
screen_width = 480
screen_height = 800
pygame.display.set_caption("飞机大战")
screen = pygame.display.set_mode((screen_width, screen_height))
# 加载背景音乐
pygame.mixer.music.load("bgm.mp3")
pygame.mixer.music.play(-1) # 播放背景音乐,-1表示循环播放
# 加载游戏音效
bullet_sound = pygame.mixer.Sound("bullet.wav")
enemy_down_sound = pygame.mixer.Sound("enemy_down.wav")
game_over_sound = pygame.mixer.Sound("game_over.wav")
# 加载游戏图片资源
background_img = pygame.image.load("background.png")
player_img = pygame.image.load("player.png")
enemy_img = pygame.image.load("enemy.png")
bullet_img = pygame.image.load("bullet.png")
cloud_img = pygame.image.load("cloud.png")
donut_img = pygame.image.load("donut.png")
# 定义游戏参数
player_speed = 5
player_rect = player_img.get_rect(center=(screen_width // 2, screen_height - 100))
bullet_speed = 8
bullet_interval = 200 # 发射子弹的时间间隔
bullet_timer = 0
enemy_speed = 2
enemy_interval = 2000 # 产生敌机的时间间隔
enemy_timer = 0
cloud_speed = 1
cloud_interval = 5000 # 产生云朵的时间间隔
cloud_timer = 0
donut_speed = 1
donut_interval = 10000 # 产生甜甜圈的时间间隔
donut_timer = 0
player_life = 3
player_bullet_type = 0 # 玩家子弹类型,0表示普通弹,1表示双排弹
score = 0
# 定义游戏状态常量
GAME_STATUS_START = 0
GAME_STATUS_PLAYING = 1
GAME_STATUS_GAME_OVER = 2
game_status = GAME_STATUS_START
# 创建字体对象
font = pygame.font.SysFont("SimHei", 30)
# 定义函数:绘制得分和生命值
def draw_text():
score_text = font.render("得分:" + str(score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
life_text = font.render("生命值:" + str(player_life), True, (255, 255, 255))
screen.blit(life_text, (screen_width - life_text.get_width() - 10, 10))
# 定义函数:绘制玩家飞机
def draw_player():
screen.blit(player_img, player_rect)
# 定义函数:发射子弹
def fire_bullet():
global player_bullet_type
if player_bullet_type == 0:
bullet_rect = bullet_img.get_rect(midbottom=(player_rect.centerx, player_rect.top))
bullets.append(bullet_rect)
else:
bullet_left_rect = bullet_img.get_rect(midbottom=(player_rect.centerx - 15, player_rect.top))
bullet_right_rect = bullet_img.get_rect(midbottom=(player_rect.centerx + 15, player_rect.top))
bullets.append(bullet_left_rect)
bullets.append(bullet_right_rect)
bullet_sound.play() # 播放发射子弹的音效
# 定义函数:绘制子弹
def draw_bullets():
for bullet_rect in bullets:
screen.blit(bullet_img, bullet_rect)
# 定义函数:移动子弹
def move_bullets():
for bullet_rect in bullets:
bullet_rect.move_ip(0, -bullet_speed)
# 移除已经离开屏幕的子弹
bullets_copy = bullets.copy()
for bullet_rect in bullets_copy:
if bullet_rect.bottom < 0:
bullets.remove(bullet_rect)
# 定义函数:产生敌机
def generate_enemy():
enemy_rect = enemy_img.get_rect(midbottom=(random.randint(0, screen_width), 0))
enemies.append(enemy_rect)
# 定义函数:绘制敌机
def draw_enemies():
for enemy_rect in enemies:
screen.blit(enemy_img, enemy_rect)
# 定义函数:移动敌机
def move_enemies():
for enemy_rect in enemies:
enemy_rect.move_ip(0, enemy_speed)
# 移除已经离开屏幕的敌机
enemies_copy = enemies.copy()
for enemy_rect in enemies_copy:
if enemy_rect.top > screen_height:
enemies.remove(enemy_rect)
# 定义函数:检测碰撞
def check_collision():
global player_life, player_bullet_type, score
# 检测子弹是否击中敌机
bullets_copy = bullets.copy()
for bullet_rect in bullets_copy:
for enemy_rect in enemies:
if bullet_rect.colliderect(enemy_rect):
bullets.remove(bullet_rect)
enemies.remove(enemy_rect)
enemy_down_sound.play() # 播放敌机被击中的音效
score += 10
# 检测敌机是否撞到玩家
for enemy_rect in enemies:
if enemy_rect.colliderect(player_rect):
enemies.remove(enemy_rect)
player_life -= 1
if player_life == 0:
game_over()
# 检测玩家是否吃到甜甜圈
donuts_copy = donuts.copy()
for donut_rect in donuts_copy:
if donut_rect.colliderect(player_rect):
donuts.remove(donut_rect)
player_bullet_type = 1
pygame.time.set_timer(pygame.USEREVENT + 2, 5000) # 设置定时器,5秒后恢复普通弹
break
# 定义函数:产生云朵
def generate_cloud():
cloud_rect = cloud_img.get_rect(midbottom=(random.randint(0, screen_width), 0))
clouds.append(cloud_rect)
# 定义函数:绘制云朵
def draw_clouds():
for cloud_rect in clouds:
screen.blit(cloud_img, cloud_rect)
# 定义函数:移动云朵
def move_clouds():
for cloud_rect in clouds:
cloud_rect.move_ip(0, cloud_speed)
# 移除已经离开屏幕的云朵
clouds_copy = clouds.copy()
for cloud_rect in clouds_copy:
if cloud_rect.top > screen_height:
clouds.remove(cloud_rect)
# 定义函数:产生甜甜圈
def generate_donut():
donut_rect = donut_img.get_rect(midbottom=(random.randint(0, screen_width), 0))
donuts.append(donut_rect)
# 定义函数:绘制甜甜圈
def draw_donuts():
for donut_rect in donuts:
screen.blit(donut_img, donut_rect)
# 定义函数:移动甜甜圈
def move_donuts():
for donut_rect in donuts:
donut_rect.move_ip(0, donut_speed)
# 移除已经离开屏幕的甜甜圈
donuts_copy = donuts.copy()
for donut_rect in donuts_copy:
if donut_rect.top > screen_height:
donuts.remove(donut_rect)
# 定义函数:游戏结束
def game_over():
global game_status
game_status = GAME_STATUS_GAME_OVER
game_over_sound.play() # 播放游戏结束的音效
# 游戏主循环
bullets = []
enemies = []
clouds = []
donuts = []
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE and game_status == GAME_STATUS_PLAYING:
if pygame.time.get_ticks() - bullet_timer >= bullet_interval:
fire_bullet()
bullet_timer = pygame.time.get_ticks()
elif event.key == pygame.K_RETURN and game_status != GAME_STATUS_PLAYING:
# 开始游戏或重新开始游戏
bullets.clear()
enemies.clear()
clouds.clear()
donuts.clear()
player_life = 3
player_bullet_type = 0
score = 0
player_rect = player_img.get_rect(center=(screen_width // 2, screen_height - 100))
game_status = GAME_STATUS_PLAYING
pygame.time.set_timer(pygame.USEREVENT + 1, enemy_interval) # 设置定时器,产生敌机
pygame.time.set_timer(pygame.USEREVENT + 3, cloud_interval) # 设置定时器,产生云朵
pygame.time.set_timer(pygame.USEREVENT + 4, donut_interval) # 设置定时器,产生甜甜圈
elif event.type == pygame.USEREVENT + 1: # 产生敌机
generate_enemy()
elif event.type == pygame.USEREVENT + 2: # 恢复普通弹
player_bullet_type = 0
elif event.type == pygame.USEREVENT + 3: # 产生云朵
generate_cloud()
elif event.type == pygame.USEREVENT + 4: # 产生甜甜圈
generate_donut()
# 更新游戏状态
if game_status == GAME_STATUS_PLAYING:
pressed_keys = pygame.key.get_pressed()
if pressed_keys[pygame.K_UP] or pressed_keys[pygame.K_w]:
player_rect.move_ip(0, -player_speed)
if pressed_keys[pygame.K_DOWN] or pressed_keys[pygame.K_s]:
player_rect.move_ip(0, player_speed)
if pressed_keys[pygame.K_LEFT] or pressed_keys[pygame.K_a]:
player_rect.move_ip(-player_speed, 0)
if pressed_keys[pygame.K_RIGHT] or pressed_keys[pygame.K_d]:
player_rect.move_ip(player_speed, 0)
# 限制玩家飞机在屏幕范围内
if player_rect.left < 0:
player_rect.left = 0
elif player_rect.right > screen_width:
player_rect.right = screen_width
if player_rect.top < 0:
player_rect.top = 0
elif player_rect.bottom > screen_height:
player_rect.bottom = screen_height
move_bullets()
move_enemies()
move_clouds()
move_donuts()
check_collision()
# 绘制游戏界面
screen.blit(background_img, (0, 0))
draw_text()
draw_player()
draw_bullets()
draw_enemies()
draw_clouds()
draw_donuts()
if game_status == GAME_STATUS_START:
# 绘制开始界面
start_text = font.render("按回车键开始游戏", True, (255, 255, 255))
screen.blit(start_text, ((screen_width - start_text.get_width()) // 2, screen_height // 2))
elif game_status == GAME_STATUS_GAME_OVER:
# 绘制游戏结束界面
game_over_text = font.render("游戏结束,得分:" + str(score), True, (255, 255, 255))
screen.blit(game_over_text, ((screen_width - game_over_text.get_width()) // 2, screen_height // 2))
restart_text = font.render("按回车键重新开始游戏", True, (255, 255, 255))
screen.blit(restart_text, ((screen_width - restart_text.get_width()) // 2, screen_height // 2 + 50))
pygame.display.update()
clock.tick(60)
```
注意:在执行示例代码前,需要将游戏图片资源、背景音乐和音效资源准备好,并且文件名需要与代码中的相同。
阅读全文
相关推荐
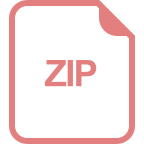
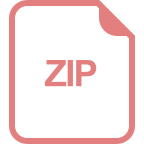
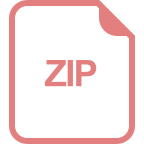




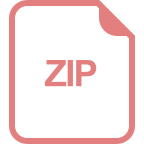