c语言读取两个共享内存中的JSON文件,并将其重新合并为一个新的JSON格式存入另一个共享内存
时间: 2024-02-05 16:12:11 浏览: 137
首先,需要使用共享内存的相关函数将两个JSON文件读入到两个不同的共享内存中,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHMSZ 1024
int main()
{
int shmid1, shmid2;
key_t key1 = 1234; // 第一个共享内存的键值
key_t key2 = 5678; // 第二个共享内存的键值
char *shm1, *shm2;
char *json1 = "{\"name\": \"Alice\", \"age\": 20}"; // 第一个JSON文件
char *json2 = "{\"name\": \"Bob\", \"age\": 25}"; // 第二个JSON文件
// 创建第一个共享内存
if ((shmid1 = shmget(key1, SHMSZ, IPC_CREAT | 0666)) < 0) {
perror("shmget");
exit(1);
}
// 将第一个共享内存连接到当前进程的地址空间
if ((shm1 = shmat(shmid1, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 将第一个JSON文件复制到第一个共享内存中
strncpy(shm1, json1, strlen(json1));
// 创建第二个共享内存
if ((shmid2 = shmget(key2, SHMSZ, IPC_CREAT | 0666)) < 0) {
perror("shmget");
exit(1);
}
// 将第二个共享内存连接到当前进程的地址空间
if ((shm2 = shmat(shmid2, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 将第二个JSON文件复制到第二个共享内存中
strncpy(shm2, json2, strlen(json2));
return 0;
}
```
然后,需要使用JSON库解析两个共享内存中的JSON文件,并将其合并为一个新的JSON格式,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <cJSON.h>
#define SHMSZ 1024
int main()
{
int shmid1, shmid2, shmid3;
key_t key1 = 1234; // 第一个共享内存的键值
key_t key2 = 5678; // 第二个共享内存的键值
key_t key3 = 9012; // 新的共享内存的键值
char *shm1, *shm2, *shm3;
cJSON *root1, *root2, *root3;
// 创建第一个共享内存
if ((shmid1 = shmget(key1, SHMSZ, IPC_CREAT | 0666)) < 0) {
perror("shmget");
exit(1);
}
// 将第一个共享内存连接到当前进程的地址空间
if ((shm1 = shmat(shmid1, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 创建第二个共享内存
if ((shmid2 = shmget(key2, SHMSZ, IPC_CREAT | 0666)) < 0) {
perror("shmget");
exit(1);
}
// 将第二个共享内存连接到当前进程的地址空间
if ((shm2 = shmat(shmid2, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 解析第一个JSON文件
root1 = cJSON_Parse(shm1);
// 解析第二个JSON文件
root2 = cJSON_Parse(shm2);
// 合并两个JSON对象
root3 = cJSON_CreateObject();
cJSON_AddItemToObject(root3, "person1", root1);
cJSON_AddItemToObject(root3, "person2", root2);
// 将新的JSON对象转换为字符串
char *json3 = cJSON_Print(root3);
// 创建新的共享内存
if ((shmid3 = shmget(key3, SHMSZ, IPC_CREAT | 0666)) < 0) {
perror("shmget");
exit(1);
}
// 将新的共享内存连接到当前进程的地址空间
if ((shm3 = shmat(shmid3, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 将新的JSON字符串复制到新的共享内存中
strncpy(shm3, json3, strlen(json3));
return 0;
}
```
最后,需要使用共享内存的相关函数将新的JSON文件从共享内存中读取出来,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHMSZ 1024
int main()
{
int shmid3;
key_t key3 = 9012; // 新的共享内存的键值
char *shm3;
// 获取新的共享内存的ID
if ((shmid3 = shmget(key3, SHMSZ, 0666)) < 0) {
perror("shmget");
exit(1);
}
// 将新的共享内存连接到当前进程的地址空间
if ((shm3 = shmat(shmid3, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
// 打印新的JSON文件
printf("%s\n", shm3);
return 0;
}
```
阅读全文
相关推荐
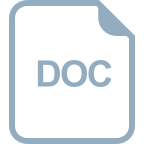
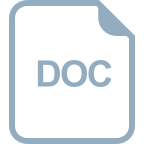
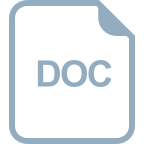
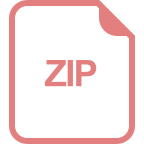
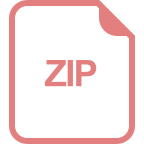
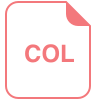
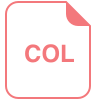
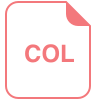
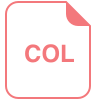
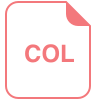
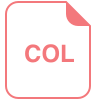
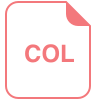
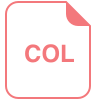
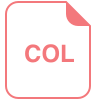

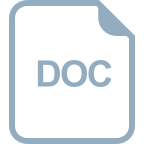
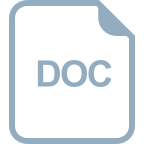
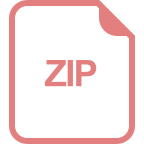
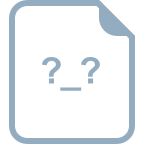