c语言使用函数完成输入最多10个大小写字符,以回车符结束输入,输出其中的大写字母,
时间: 2024-05-27 14:14:03 浏览: 59
#include <stdio.h>
void printUpperCase(char *str) {
for(int i = 0; str[i] != '\0'; i++) {
if(str[i] >= 'A' && str[i] <= 'Z') {
printf("%c ", str[i]);
}
}
}
int main() {
char str[11]; // 最多10个字符,加上最后一个'\0'占用11个位置
int i = 0;
char c;
printf("请输入最多10个大小写字符,以回车符结束:\n");
while((c = getchar()) != '\n' && i < 10) {
str[i++] = c;
}
str[i] = '\0'; // 将最后一个位置赋为'\0'
printf("大写字母为:");
printUpperCase(str);
printf("\n");
return 0;
}
相关问题
输入一个以回车符结束的字符串,统计并输出其中大写辅音字母的个数。 相关知识 21个辅音字母大小写: Bb、Cc、Dd、Ff、Gg、Hh、Jj、Kk、Ll、Mm、Nn、Pp、Qq、Rr、Ss、Tt、Vv、Ww、Xx、Yy、Zz。
可以使用循环遍历字符串中的每一个字符,判断是否为大写辅音字母,如果是则计数器加一。可以使用C语言中的字符函数isupper()和isalpha()来判断是否为大写字母和字母。具体实现代码如下:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int count = 0;
printf("请输入一个字符串:");
fgets(str, 100, stdin); // 使用fgets()函数输入字符串
for (int i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i]) && isupper(str[i])) { // 判断是否为大写字母
switch (str[i]) { // 判断是否为辅音字母
case 'B':
case 'C':
case 'D':
case 'F':
case 'G':
case 'H':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
count++;
break;
default:
break;
}
}
}
printf("大写辅音字母的个数为:%d\n", count);
return 0;
}
```
C语言用户从键盘输入一行字符,分别统计其中英文字母大小写均含、数字字符和其他字符的个数并输出不计回车符号
在C语言中,你可以使用`ctype.h`库提供的函数来检查每个字符的类别,并统计各种类型的字符。下面是一个简单的程序示例,它会提示用户输入一行字符,然后计算字母、数字和其它字符的数量:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int alpha_count = 0, digit_count = 0, other_count = 0;
char input[100];
printf("请输入一行字符(不含回车符):");
fgets(input, sizeof(input), stdin);
// 删除末尾的换行符 '\n'
input[strcspn(input, "\n")] = '\0';
for (char c : input) {
if (isalpha(c)) { // 检查是否为字母
alpha_count++;
} else if (isdigit(c)) { // 检查是否为数字
digit_count++;
} else { // 其他字符(包括空格、标点等)
other_count++;
}
}
printf("大写字母:%d\n", alpha_count);
printf("小写字母:%d\n", alpha_count); // 因为ASCII码中大写和小写只相差一位
printf("数字字符:%d\n", digit_count);
printf("其他字符:%d\n", other_count);
return 0;
}
```
在这个程序中,我们使用了`isalpha()`函数检测字母,`isdigit()`函数检测数字。注意,由于ASCII码的特点,大写字母和小写字母的检测在这里可以合并,因为它们只是编码值上相差很小。
阅读全文
相关推荐
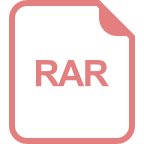

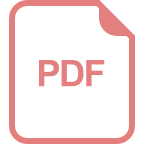
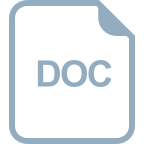
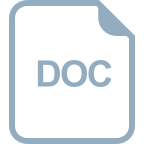
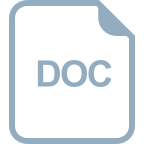
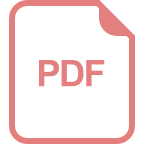
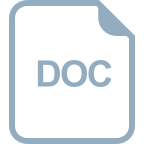
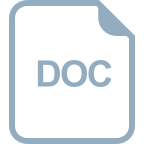
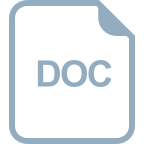
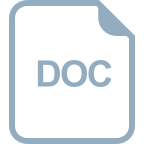
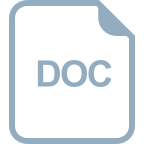
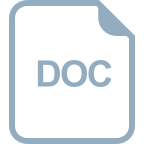
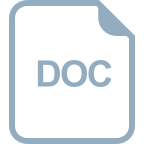
