java编写窗体应用程序,安排一个文本框、一个按钮和一个标签,从文本框录入一个数字(0~9),点击按钮将其对应的英文单词(如:zero,one等)显示在标签中。
时间: 2023-06-07 10:06:47 浏览: 132
好的,这是一个非常基础的GUI编程问题,以下是java实现的一种方式:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class NumberToWordConverter extends JFrame implements ActionListener {
private JLabel label;
private JTextField textField;
private JButton button;
public NumberToWordConverter() {
setTitle("数字转英文单词");
setLayout(new FlowLayout());
label = new JLabel("输入数字:");
add(label);
textField = new JTextField(5);
add(textField);
button = new JButton("转换");
button.addActionListener(this);
add(button);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String input = textField.getText();
int num = Integer.parseInt(input);
String[] words = {"zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"};
if (num >= 0 && num <= 9) {
label.setText(words[num]);
} else {
label.setText("输入有误!");
}
}
public static void main(String[] args) {
new NumberToWordConverter();
}
}
```
上述代码创建了一个NumberToWordConverter类,继承了JFrame类,并实现了ActionListener接口。其中,包括一个标签、一个文本框和一个按钮,并在按钮被点击时触发actionPerformed方法进行事件处理。在方法中,根据输入的数字选择对应英文单词,并在标签中显示结果。此外,还增加了输入检查,如果输入不在0~9范围内,标签会显示“输入有误!”提示信息。
阅读全文
相关推荐



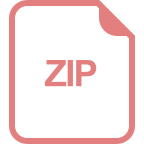
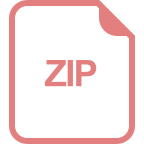
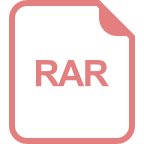
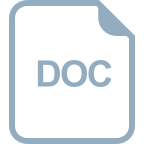







