QT中使用9个线程读文件数据
时间: 2024-05-04 16:20:10 浏览: 86
在QT中使用多线程读取文件数据可以提高程序的效率。以下是一个使用9个线程读取文件数据的示例:
首先,在主线程中定义线程数和文件路径:
```cpp
int threadNum = 9; // 线程数
QString filePath = "your_file_path"; // 文件路径
```
然后,定义一个继承自QThread的类,用于读取文件数据:
```cpp
class FileReader : public QThread {
Q_OBJECT
public:
explicit FileReader(QObject *parent = nullptr) : QThread(parent) {}
void run() override {
QFile file(filePath);
if (!file.open(QIODevice::ReadOnly | QIODevice::Text)) {
qDebug() << "Failed to open file";
return;
}
QTextStream in(&file);
QString line;
int lineNum = 0;
while (!in.atEnd()) {
line = in.readLine();
if (lineNum % threadNum == threadIndex) { // 读取该线程负责的数据
// 处理读取到的数据
}
lineNum++;
}
}
signals:
void finished();
private:
int threadIndex; // 线程编号
int threadNum; // 线程数
QString filePath; // 文件路径
};
```
在该类中,我们重写了run()函数,用于读取文件数据并处理。在run()函数中,我们首先打开文件并使用QTextStream读取数据。然后,我们遍历文件中的每一行,并根据线程数和线程编号确定该线程负责读取哪些数据。最后,我们将读取到的数据进行处理。我们还定义了一个finished()信号,在线程读取完成后发出。
接下来,在主线程中创建9个线程并启动它们:
```cpp
QList<FileReader*> threadList;
for (int i = 0; i < threadNum; i++) {
FileReader *thread = new FileReader();
thread->threadIndex = i;
thread->threadNum = threadNum;
thread->filePath = filePath;
connect(thread, SIGNAL(finished()), thread, SLOT(deleteLater()));
threadList.append(thread);
thread->start();
}
```
在这里,我们创建了9个线程,并将线程编号、线程数和文件路径设置为我们之前定义的值。我们还将每个线程的finished()信号连接到该线程的deleteLater()槽函数,确保线程完成后能够正确释放资源。最后,我们启动每个线程。
以上就是在QT中使用9个线程读取文件数据的示例。需要注意的是,在实际使用中,还需要考虑线程间的数据同步和线程池的使用等问题。
阅读全文
相关推荐
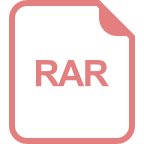



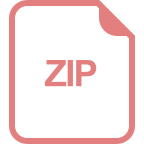
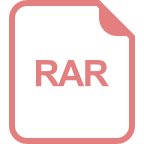
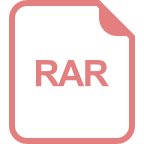
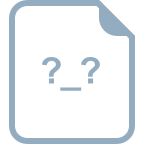
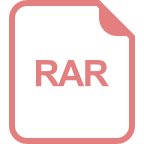
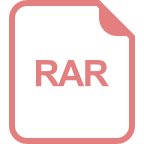
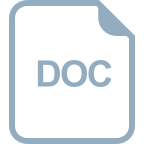
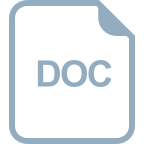




