在主线程打开文件 ,启用子线程读取文件内容 qt
时间: 2024-03-09 13:46:23 浏览: 21
在Qt中,我们可以在主线程中打开文件,然后在一个子线程中读取文件内容。具体步骤如下:
1. 创建一个继承自`QThread`的子类,例如`FileReaderThread`。
2. 在`FileReaderThread`类中定义一个函数,用于设置要读取的文件路径。
```c++
void FileReaderThread::setFilePath(const QString& filePath)
{
m_filePath = filePath;
}
```
3. 在`FileReaderThread`类中重载`run()`函数,该函数中实现文件读取的逻辑。
```c++
void FileReaderThread::run()
{
QFile file(m_filePath);
if (file.open(QIODevice::ReadOnly))
{
QByteArray data = file.readAll();
emit fileContentReady(data);
file.close();
}
}
```
在上面的代码中,我们首先打开文件,然后读取文件内容到`QByteArray`中,并通过信号`fileContentReady`将读取到的数据发送到主线程。
4. 在主线程中创建一个`FileReaderThread`的实例,并连接`fileContentReady`信号到槽函数。
```c++
FileReaderThread* fileReaderThread = new FileReaderThread(this);
connect(fileReaderThread, SIGNAL(fileContentReady(QByteArray)), this, SLOT(onFileContentReady(QByteArray)));
fileReaderThread->start();
```
5. 在`onFileOpen()`槽函数中打开文件,并将文件路径设置给`FileReaderThread`实例。
```c++
void MainWindow::onFileOpen()
{
QString filePath = QFileDialog::getOpenFileName(this, tr("Open File"), "", tr("Text Files (*.txt)"));
if (!filePath.isEmpty())
{
m_fileReaderThread->setFilePath(filePath);
}
}
```
6. 在`onFileContentReady()`槽函数中处理读取到的文件内容,例如:更新UI界面或者进行其他操作。
```c++
void MainWindow::onFileContentReady(QByteArray data)
{
ui->textEdit->setText(QString(data));
}
```
在上述代码中,我们首先创建了一个`FileReaderThread`的实例,并连接了`fileContentReady`信号到槽函数`onFileContentReady()`。然后我们在`onFileOpen()`函数中打开文件,并将文件路径设置给`FileReaderThread`实例。最后,在`onFileContentReady()`函数中处理读取到的文件内容。
注意:上述代码仅为示例,具体实现方式还需要根据你的具体需求进行调整。
相关推荐
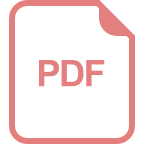














