qt从文件解析json
时间: 2023-07-22 18:40:16 浏览: 123
在Qt中,从文件解析JSON可以使用Qt自带的QFile和QJsonDocument类。下面是一个简单的示例代码:
```cpp
#include <QFile>
#include <QJsonDocument>
#include <QJsonObject>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QFile file("example.json");
if(!file.open(QIODevice::ReadOnly | QIODevice::Text))
{
qDebug() << "Failed to open file.";
return -1;
}
QByteArray jsonData = file.readAll();
QJsonDocument jsonDocument = QJsonDocument::fromJson(jsonData);
if(!jsonDocument.isNull())
{
if(jsonDocument.isObject())
{
QJsonObject jsonObject = jsonDocument.object();
if(jsonObject.contains("name"))
{
QJsonValue nameValue = jsonObject.value("name");
if(nameValue.isString())
{
QString name = nameValue.toString();
qDebug() << "name:" << name;
}
}
if(jsonObject.contains("age"))
{
QJsonValue ageValue = jsonObject.value("age");
if(ageValue.isDouble())
{
int age = ageValue.toInt();
qDebug() << "age:" << age;
}
}
if(jsonObject.contains("city"))
{
QJsonValue cityValue = jsonObject.value("city");
if(cityValue.isString())
{
QString city = cityValue.toString();
qDebug() << "city:" << city;
}
}
}
}
file.close();
return a.exec();
}
```
代码中,首先定义了一个文件名为example.json的文件,然后通过QFile::open()方法打开文件并读取文件中的JSON数据。接着,通过QJsonDocument::fromJson()方法将JSON数据转换为QJsonDocument对象。判断QJsonDocument对象是否为空和是否为对象类型,如果是,就通过QJsonObject类获取JSON对象中的属性值,判断属性值的类型后,获取其值并输出。最后,关闭文件。
阅读全文
相关推荐
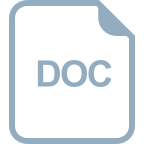
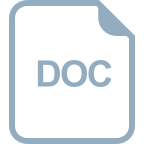
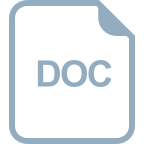
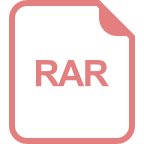
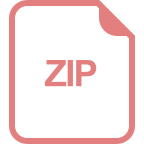
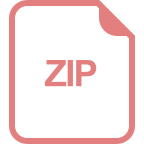
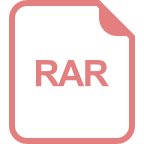
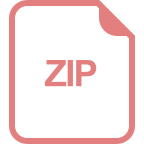
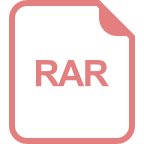
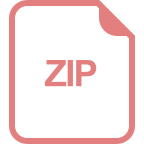
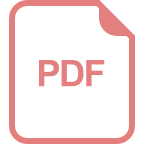
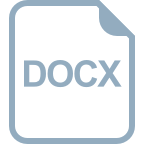
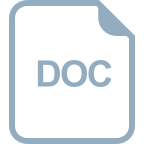





