怎么用flask调用数据库
时间: 2023-08-02 18:34:15 浏览: 107
下面是使用 Flask 框架调用 SQLite 数据库的一些基本步骤:
1. 安装 Flask 和 SQLite3。您可以使用 pip 包管理器来安装这些库。
```python
pip install flask
pip install sqlite3
```
2. 创建一个数据库。您可以使用 SQLite3 命令行工具或其他工具来创建 SQLite 数据库。
3. 在 Flask 应用程序中导入 Flask 和 SQLite3 模块。
```python
from flask import Flask, render_template, request
import sqlite3
```
4. 创建 Flask 应用程序。
```python
app = Flask(__name__)
```
5. 设置数据库连接和游标。
```python
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
```
6. 创建一个视图函数,该函数将从数据库中检索数据并将其呈现到 Flask 应用程序的模板中。
```python
@app.route('/')
def index():
cursor.execute("SELECT * FROM mytable")
rows = cursor.fetchall()
return render_template('index.html', rows=rows)
```
7. 创建一个 HTML 模板,用于呈现从数据库中检索到的数据。
```html
<!DOCTYPE html>
<html>
<head>
<title>Flask SQLite</title>
</head>
<body>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
{% for row in rows %}
<tr>
<td>{{ row[0] }}</td>
<td>{{ row[1] }}</td>
<td>{{ row[2] }}</td>
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
```
8. 运行 Flask 应用程序。
```python
if __name__ == '__main__':
app.run(debug=True)
```
以上就是基本的 Flask 调用 SQLite 数据库的步骤。当然,您可以根据自己的需要进行修改和定制。
阅读全文
相关推荐
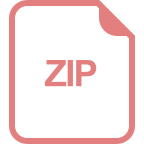



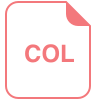


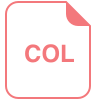
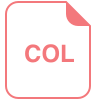







