1. 编写一个程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数
时间: 2024-03-05 10:49:45 浏览: 124
以下是一个简单的 Python 程序,可以实现您的需求:
``` python
import os
import struct
from Crypto.Cipher import AES
# 加密函数
def encrypt(plaintext, key):
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext.encode())
return cipher.nonce + tag + ciphertext
# 解密函数
def decrypt(ciphertext, key):
nonce, tag, ciphertext = ciphertext[:16], ciphertext[16:32], ciphertext[32:]
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
return plaintext.decode()
# 保存文件函数
def save_file(filename, plaintext, key):
# 加密明文
ciphertext = encrypt(plaintext, key)
# 将文件结构和密文合并
data = struct.pack("16s16s%ds" % len(ciphertext), key, ciphertext)
# 写入文件
with open(filename, "wb") as f:
f.write(data)
# 加载文件函数
def load_file(filename, key, max_attempts=3):
# 读取文件
with open(filename, "rb") as f:
data = f.read()
# 解析文件结构
key2, ciphertext = struct.unpack("16s%ds" % (len(data) - 16), data)
# 验证密钥
for i in range(max_attempts):
if key2 == key:
# 解密密文
return decrypt(ciphertext, key)
else:
# 密钥错误,重新输入
key = input("Invalid password. Please try again (%d/%d): " % (i+1, max_attempts)).encode()
# 密钥错误次数过多,退出程序
print("Too many attempts. Exiting...")
exit()
# 测试程序
if __name__ == "__main__":
filename = "姓名.dat"
plaintext = "Hello, World!"
key = b"my_secret_key_123"
save_file(filename, plaintext, key)
ciphertext = load_file(filename, key)
print(ciphertext)
```
程序使用了 PyCrypto 库中的 AES 加密算法,要求在运行程序前先安装该库。程序包括以下功能:
1. `encrypt(plaintext, key)`:加密函数,使用给定的密钥将明文加密成密文。
2. `decrypt(ciphertext, key)`:解密函数,使用给定的密钥将密文解密成明文。
3. `save_file(filename, plaintext, key)`:保存文件函数,将给定的明文使用给定的密钥加密后保存到文件中。
4. `load_file(filename, key, max_attempts=3)`:加载文件函数,从文件中读取密文并使用给定的密钥解密,要求在解密前输入密码并验证,最多允许输入 `max_attempts` 次密码。
程序运行后会将明文字符串加密并保存到名为“姓名.dat”的文件中,然后重新读取该文件并解密密文。如果输入的密码与保存时使用的密码不同,程序会提示重新输入,最多允许输入 `max_attempts` 次密码,超过次数后程序会退出。
阅读全文
相关推荐
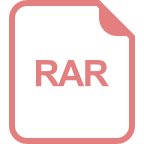
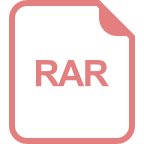




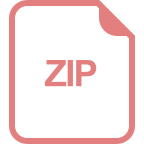
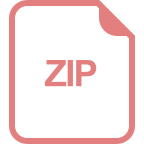
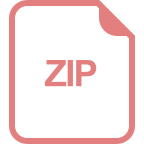
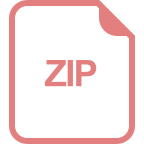
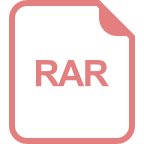
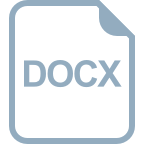
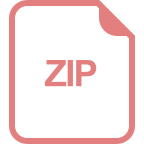
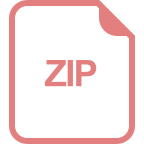