编程实现 a) 调用 pipe()创建无名管道 b) 调用 fork 创建一个子进程 c) 子进程 - 用 signal( )安装信号 SIGALRM 在 SIGALRM 的信号处理函数内执行下列操作: 打印出 pid 和接收到的信号值 向管道内写入所有的小写字母,打印出进程号及成功写入的字节数 - 用 signal( )安装信号 SIGINT 在 SIGINT 的信号处理函数内执行下列操作: 打印出 pid 和接收到的信号值 重新安装信号 SIGINT,采用默认方式处理该信号 - 设置一个定时器,2s 后产生 SIGALRM 信号 - 进入 while(1)循环,每隔 1s 打印出一行” ...child is waiting” d) 父进程 - 打印出进程号 - 从管道读出数据,每次读 8 个字符,直到读出管道中的所有内容 打印出每次读出的字节数及读出的内容 - 向子进程发送信号 SIGINT - 等待 1s - 再次向子进程发送信号 SIGINT - 等待子进程结束 - 父进程退出 请参考以下格式输出:(具体的执行顺序、pid、...child is waiting 打印的次数可以不同) parent pid:221 ...child is waiting ...child is waiting pid:222, sig=14 send 26 bytes receive 8 bytes, abcdefgh ...child is waiting receive 8 bytes, ijklmnop receive 8 bytes, qrstuvwx receive 2 bytes, yz pid:222, sig=2 ...child is waiting
时间: 2024-03-03 21:48:44 浏览: 40
以下是实现该功能的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <sys/types.h>
#include <sys/wait.h>
#define BUFFER_SIZE 26
int pipe_fd[2]; // 管道文件描述符数组
pid_t child_pid; // 子进程的进程ID
void sig_alrm_handler(int signo) {
printf("pid:%d, sig=%d\n", getpid(), signo);
// 向管道内写入所有的小写字母
char buffer[BUFFER_SIZE];
for (int i = 0; i < BUFFER_SIZE; i++) {
buffer[i] = 'a' + i;
}
int n = write(pipe_fd[1], buffer, BUFFER_SIZE);
printf("pid:%d, send %d bytes\n", getpid(), n);
}
void sig_int_handler(int signo) {
printf("pid:%d, sig=%d\n", getpid(), signo);
// 重新安装信号 SIGINT,采用默认方式处理该信号
signal(SIGINT, SIG_DFL);
}
int main() {
// 调用 pipe() 创建无名管道
if (pipe(pipe_fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
// 调用 fork() 创建一个子进程
if ((child_pid = fork()) == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (child_pid == 0) { // 子进程
// 用 signal( ) 安装信号 SIGALRM
signal(SIGALRM, sig_alrm_handler);
// 用 signal( ) 安装信号 SIGINT
signal(SIGINT, sig_int_handler);
// 设置一个定时器,2s 后产生 SIGALRM 信号
alarm(2);
// 进入 while(1) 循环,每隔 1s 打印出一行"...child is waiting"
while (1) {
printf("...child is waiting\n");
sleep(1);
}
} else { // 父进程
// 打印出进程号
printf("parent pid:%d\n", getpid());
// 从管道读出数据,每次读 8 个字符,直到读出管道中的所有内容
char buffer[BUFFER_SIZE];
int n;
while ((n = read(pipe_fd[0], buffer, BUFFER_SIZE)) > 0) {
printf("receive %d bytes, %.*s\n", n, n, buffer);
}
// 向子进程发送信号 SIGINT
kill(child_pid, SIGINT);
// 等待 1s
sleep(1);
// 再次向子进程发送信号 SIGINT
kill(child_pid, SIGINT);
// 等待子进程结束
waitpid(child_pid, NULL, 0);
// 父进程退出
exit(EXIT_SUCCESS);
}
return 0;
}
```
相关推荐
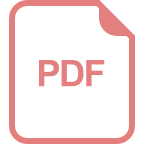
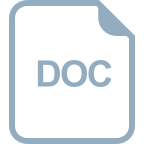
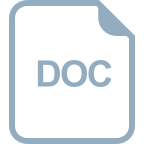















