用Python写一个五子棋程序
时间: 2023-11-30 19:03:38 浏览: 77
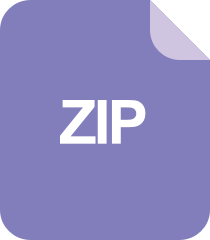
基于Python的五子棋程序代码.zip

好的,以下是一个简单的五子棋程序的示例代码:
```python
import numpy as np
# 初始化棋盘
def init_board():
board = np.zeros((15, 15), dtype=int)
return board
# 落子
def put_stone(board, player, position):
board[position[0], position[1]] = player
# 判断是否有五子连珠
def is_win(board, player):
# 横向判断
for i in range(15):
for j in range(11):
if board[i][j] == player and board[i][j+1] == player and board[i][j+2] == player and board[i][j+3] == player and board[i][j+4] == player:
return True
# 竖向判断
for i in range(11):
for j in range(15):
if board[i][j] == player and board[i+1][j] == player and board[i+2][j] == player and board[i+3][j] == player and board[i+4][j] == player:
return True
# 左上到右下斜向判断
for i in range(11):
for j in range(11):
if board[i][j] == player and board[i+1][j+1] == player and board[i+2][j+2] == player and board[i+3][j+3] == player and board[i+4][j+4] == player:
return True
# 右上到左下斜向判断
for i in range(11):
for j in range(4, 15):
if board[i][j] == player and board[i+1][j-1] == player and board[i+2][j-2] == player and board[i+3][j-3] == player and board[i+4][j-4] == player:
return True
return False
# 判断是否平局
def is_draw(board):
return np.count_nonzero(board) == 15 * 15
# 主函数
def main():
# 初始化棋盘
board = init_board()
# 玩家1为黑子,玩家2为白子
players = [1, 2]
# 初始化当前玩家为玩家1
current_player = 0
# 游戏循环
while True:
# 让当前玩家落子
position_str = input("Player %d, please enter your position (e.g. 1,1): " % (current_player+1))
position = tuple(map(int, position_str.split(',')))
put_stone(board, players[current_player], position)
# 判断游戏是否结束
if is_win(board, players[current_player]):
print("Player %d wins!" % (current_player+1))
break
if is_draw(board):
print("The game is a draw!")
break
# 切换到下一个玩家
current_player = (current_player + 1) % 2
# 打印当前棋盘
print(board)
if __name__ == '__main__':
main()
```
这个程序使用了 NumPy 库来管理棋盘,使用了一个 15x15 的二维数组来表示棋盘,其中 0 表示空格,1 表示黑子,2 表示白子。程序通过循环让两个玩家轮流落子,并且在每次落子后判断是否有五子连珠或者是否平局。如果游戏结束,程序会打印出胜者或者平局的信息。
阅读全文
相关推荐
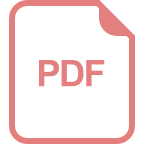












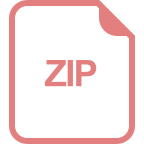
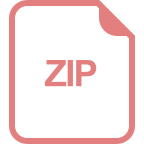