String returnCode = String.valueOf(resultMap.get("code"));解释一下
时间: 2024-04-21 16:24:12 浏览: 13
这段代码的作用是从 `resultMap` 中获取名为 "code" 的值,并将其转换为字符串类型赋值给 `returnCode` 变量。`resultMap` 是前面提到的通过解析 JSON 字符串得到的 `Map` 对象。
在这个例子中,假设 `resultMap` 是一个包含键值对的 Map 对象,其中包含一个名为 "code" 的键。通过调用 `resultMap.get("code")` 方法,可以获取到与键 "code" 相对应的值。由于 `resultMap` 的值类型是 Object,因此使用 `String.valueOf()` 方法将其转换为字符串类型。最后,将转换后的字符串值赋给 `returnCode` 变量,以便后续使用。
相关问题
1 == Integer.parseInt(resultMap.get("success").toString()) 怎么取反
要将 `1 == Integer.parseInt(resultMap.get("success").toString())` 的结果取反,可以使用逻辑非运算符 "!",例如:
```
if (!(1 == Integer.parseInt(resultMap.get("success").toString()))) {
// do something
}
```
这样可以在 `resultMap.get("success")` 不是整数类型的1时执行 "do something" 中的代码。另外,需要注意的是,在 `resultMap.get("success")` 为 null 或者不包含 "success" 这个键时,上述代码也会执行 "do something" 中的代码。如果需要避免这种情况,可以在执行条件语句之前先判断 `resultMap` 是否包含 "success" 这个键,例如:
```
if (resultMap.containsKey("success") && !(1 == Integer.parseInt(resultMap.get("success").toString()))) {
// do something
}
```
这样可以避免空指针异常和键不存在的问题。
public List<String> getPermissionByType() { String userIdString = request.getHeader(Consts.USER_ID); Long userId = Long.valueOf(userIdString); // 先去查 redis里面有没有对应的 key String key = "DataPermission:" + userIdString; Map<String, List<String>> userDataPermissionMap = (Map<String, List<String>>) redisTemplate.opsForValue().get(key); List<String> result = new ArrayList<>(); // 当前用户权限类型 String mallType = menuInfoService.getMallType(userIdString); String type; if (MallPermissionEnums.NO_PERMISSION.equalsStr(mallType)){ return new ArrayList<>(); } else if (MallPermissionEnums.BOUTIQUE.equalsStr(mallType)){ type = AccountAccessTypeEnums.BOUTIQUE_SO_TYPE.getCode(); } else if (MallPermissionEnums.AFTER_SALES.equalsStr(mallType)){ type = AccountAccessTypeEnums.SUB_ORDER_TYPE.getCode(); } else { type = AccountAccessTypeEnums.ALL.getCode(); } if (ObjectUtils.isEmpty(userDataPermissionMap) || ObjectUtils.isEmpty(userDataPermissionMap.values())){ Map<String, List<String>> resultMap = setUserDataPermissionToRedis(key,userId); if (AccountAccessTypeEnums.ALL.getCode().equals(type)){ resultMap.values().forEach(result::addAll); return result; } else { return resultMap.get(type); } } else if (AccountAccessTypeEnums.ALL.getCode().equals(type)){ userDataPermissionMap.values().forEach(result::addAll); return result; } else { return userDataPermissionMap.get(type); } }对以上代码编写单元测试
Sure! Here's an example of how you can write a unit test for the given code snippet using JUnit and Mockito:
```java
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.springframework.data.redis.core.RedisTemplate;
import java.util.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.mockito.Mockito.when;
public class PermissionTest {
@Mock
private RedisTemplate<String, Object> redisTemplate;
@Mock
private MenuInfoService menuInfoService;
@InjectMocks
private YourClassName yourClassName;
@BeforeEach
public void setup() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testGetPermissionByType() {
// Mocking the request header
String userIdString = "123";
when(request.getHeader(Consts.USER_ID)).thenReturn(userIdString);
// Mocking the redis data
Map<String, List<String>> userDataPermissionMap = new HashMap<>();
userDataPermissionMap.put(AccountAccessTypeEnums.BOUTIQUE_SO_TYPE.getCode(), Arrays.asList("permission1", "permission2"));
when(redisTemplate.opsForValue().get("DataPermission:" + userIdString)).thenReturn(userDataPermissionMap);
// Mocking the menuInfoService.getMallType() response
when(menuInfoService.getMallType(userIdString)).thenReturn(MallPermissionEnums.BOUTIQUE.getStr());
// Calling the method to be tested
List<String> result = yourClassName.getPermissionByType();
// Asserting the result
List<String> expected = Arrays.asList("permission1", "permission2");
assertEquals(expected, result);
}
}
```
In this unit test, we use Mockito to mock the dependencies (`redisTemplate`, `menuInfoService`) and inject them into the instance of the class being tested (`yourClassName`). We then mock the necessary method calls and set the expected values for the test case. Finally, we call the method `getPermissionByType()` and assert the expected result against the actual result.
相关推荐
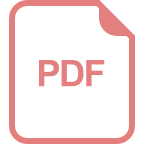
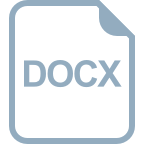













