c++把图书表抽象成一个线性表,每本图书(包括ISBN,书名,定价)作为线性表中的一个元素。在图书信息管理系统中要求实现建表、输出、查找、插入、删除等功能
时间: 2024-10-14 20:16:00 浏览: 30
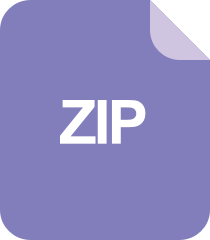
基于线性表的图书管理系统 源代码 顺序表&链表
在C++中,将图书信息抽象成一个线性表,比如可以使用数组或动态存储容器(如std::vector)来实现。每个图书记录(包含ISBN、书名和定价)都可以作为一个结构体或类的对象,存放在线性表的元素里。以下是针对这个需求的基本操作:
1. **建表** (Creating the table):
- 创建一个图书类(Book),包含成员变量表示ISBN、书名和定价。
```cpp
struct Book {
std::string ISBN;
std::string title;
double price;
};
```
或者使用`class`关键字创建类。
2. **输出** (Outputting the data):
- 定义一个函数遍历线性表并打印图书信息。
```cpp
void display(const Book* books, int size) {
for (int i = 0; i < size; ++i) {
std::cout << "ISBN: " << books[i].ISBN << ", Title: " << books[i].title << ", Price: " << books[i].price << "\n";
}
}
```
3. **查找** (Searching):
- 提供一个查找功能,通过ISBN搜索特定图书。
```cpp
bool find(Book* books, int size, const std::string& targetISBN, int& index) {
for (index = 0; index < size; ++index) {
if (books[index].ISBN == targetISBN) return true;
}
return false;
}
```
4. **插入** (Inserting):
- 添加一个函数在指定位置插入新的图书记录。
```cpp
void insert(Book*& books, int& size, int position, const Book& newBook) {
Book temp = books[size];
// Shift elements right and insert at the given position
for (int i = size; i > position; --i)
books[i] = books[i - 1];
books[position] = newBook;
++size;
}
```
5. **删除** (Deleting):
- 删除指定位置的图书记录。
```cpp
void deleteEntry(Book*& books, int& size, int position) {
if (position >= 0 && position < size) {
for (int i = position; i < size - 1; ++i)
books[i] = books[i + 1];
--size;
}
}
```
阅读全文
相关推荐
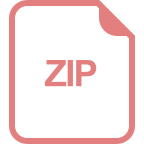


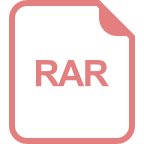
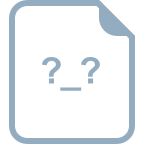
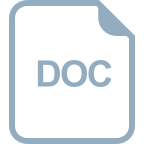








