c++给定两个字符串,在字符串A中查找字符串B删除重复的字符串并输出
时间: 2024-10-27 19:01:30 浏览: 34
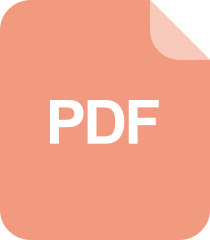
C++实现将一个字符串中的字符替换成另一个字符串的方法
在C++中,你可以通过多种方法实现这个功能。一种常见的做法是利用`std::set`容器来存储不重复的字符串。`std::set`自动去重,并按照字典序排序元素。以下是简单的步骤:
```cpp
#include <iostream>
#include <string>
#include <set>
std::string findAndRemoveDuplicates(const std::string& stringA, const std::string& stringB) {
std::set<std::string> uniqueStrings;
std::string substrings[2] = {stringB, stringA}; // 存储待搜索的两个字符串
for (const auto& s : substrings) {
size_t pos = 0;
while ((pos = stringA.find(s, pos)) != std::string::npos) {
// 删除重复部分
stringA.erase(pos, s.length());
pos += s.length();
}
}
return stringA; // 返回处理后的结果
}
int main() {
std::string strA = "hello worldhello";
std::string strB = "world";
std::cout << "Original A: " << strA << "\n";
std::string result = findAndRemoveDuplicates(strA, strB);
std::cout << "After removing duplicates in A with respect to B: " << result << "\n";
return 0;
}
```
在这个例子中,函数`findAndRemoveDuplicates`遍历`stringA`,查找与`stringB`匹配的部分并移除,直到没有更多的匹配。最后返回处理后的`stringA`。
阅读全文
相关推荐
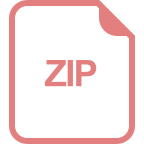
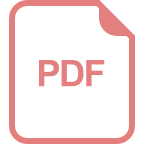















