C++给定两个字符串,在字符串A中查找字符串B遇到B时删除并输出删除后的字符串
时间: 2024-10-29 09:00:29 浏览: 20
在C++中,你可以通过迭代遍历的方式来实现这个功能。可以创建一个名为`findAndDelete()`的函数,接受两个字符串作为输入参数。以下是简单的步骤:
1. 定义两个字符串变量 `strA` 和 `strB`。
2. 使用 `std::string::find()` 函数来查找 `strB` 是否出现在 `strA` 中。如果找到,返回其起始位置;如果没有找到,返回 -1。
3. 使用一个循环,从第一个匹配的位置开始,每次找到 `strB` 就删除它,并更新 `strA`。
4. 当找不到 `strB` 时,输出删除后的 `strA`。
下面是一个简化的示例代码:
```cpp
#include <iostream>
#include <string>
void findAndDelete(std::string &strA, const std::string &strB) {
size_t pos = strA.find(strB);
while (pos != std::string::npos) {
strA.erase(pos, strB.length());
pos = strA.find(strB, pos); // 更新查找起始位置
}
std::cout << "删除后的字符串: " << strA << std::endl;
}
int main() {
std::string strA = "Hello World, this is a test.";
std::string strB = "is";
findAndDelete(strA, strB);
return 0;
}
```
运行此程序,你会看到输出类似于 "Hello World, this t." 的结果,因为 "is" 被删除了。
阅读全文
相关推荐
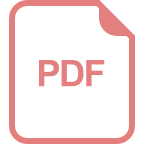
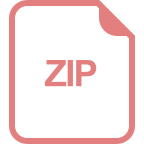
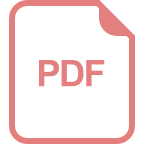















