java代码实现读取pdf每一页内容并并分别存入数据库
时间: 2024-02-23 10:02:58 浏览: 287
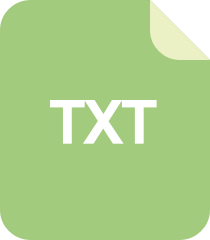
Java 读取pdf文件中的数据
要实现读取PDF文件并将每一页内容分别存入数据库,我们可以使用以下步骤:
1. 使用PDFBox库来读取PDF文件,并获取每一页的内容。
2. 使用JDBC连接数据库,并创建一个表用于存储PDF文件的内容。
3. 对于每一页的内容,将其存入数据库中的不同行中。
以下是一个示例代码,用于读取PDF文件并将每一页的内容存入MySQL数据库中:
```java
import java.io.File;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.text.PDFTextStripper;
public class PDFReader {
public static void main(String[] args) {
try {
// Load MySQL JDBC driver
Class.forName("com.mysql.jdbc.Driver");
// Connect to MySQL server
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/pdf_database", "username", "password");
// Create table for PDF content
PreparedStatement createTable = conn.prepareStatement("CREATE TABLE IF NOT EXISTS pdf_content (id INT AUTO_INCREMENT PRIMARY KEY, page_number INT, content TEXT)");
createTable.executeUpdate();
// Read the PDF file
PDDocument document = PDDocument.load(new File("example.pdf"));
// Create PDFTextStripper object to extract text
PDFTextStripper stripper = new PDFTextStripper();
// Loop through each page and extract text
for (int i = 1; i <= document.getNumberOfPages(); i++) {
stripper.setStartPage(i);
stripper.setEndPage(i);
String text = stripper.getText(document);
// Insert text into database
PreparedStatement insertContent = conn.prepareStatement("INSERT INTO pdf_content (page_number, content) VALUES (?, ?)");
insertContent.setInt(1, i);
insertContent.setString(2, text);
insertContent.executeUpdate();
}
// Close the PDF document
document.close();
// Close the database connection
conn.close();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上述示例代码中,我们使用PDFBox库中的PDDocument类来加载PDF文件,并使用PDFTextStripper类来提取每一页的内容。我们还使用JDBC来连接MySQL数据库,并使用PreparedStatement对象来执行SQL语句。在循环中,我们将每一页的内容存储到数据库中,每一页存储在数据库表中的不同行中。
阅读全文
相关推荐
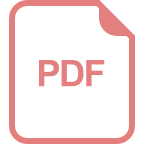
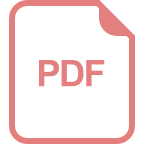
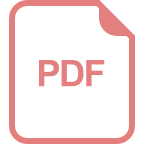
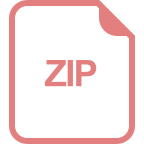
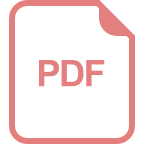
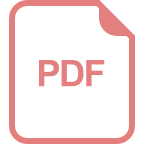
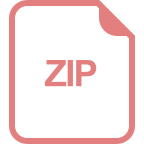
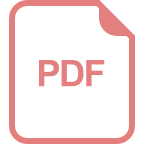
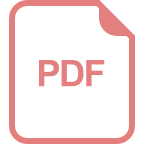
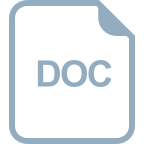
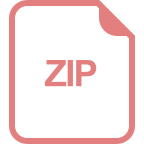
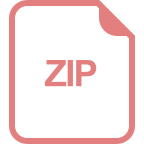
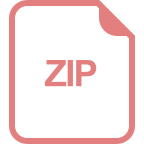