课程设计如何加入文件操作
时间: 2024-05-06 13:18:01 浏览: 5
在一个课程设计中加入文件操作,可以使得程序更加实用和完善。以下是几种文件操作的方式:
1. 读取文件内容:使用文件输入流将文件的内容读取到程序中,可以使用C++标准库中的fstream来实现。
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ifstream file("example.txt");
string line;
while (getline(file, line))
{
cout << line << endl;
}
return 0;
}
```
2. 写入文件内容:使用文件输出流将程序中的内容写入到文件中,同样可以使用C++标准库中的fstream来实现。
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ofstream file("example.txt");
if (file.is_open())
{
file << "Hello World!" << endl;
file << "This is an example." << endl;
file.close();
}
else
{
cout << "Unable to open file" << endl;
}
return 0;
}
```
3. 追加文件内容:使用文件输出流打开文件时可以使用ios::app选项来追加内容。
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ofstream file("example.txt", ios::app);
if (file.is_open())
{
file << "This is an additional line." << endl;
file.close();
}
else
{
cout << "Unable to open file" << endl;
}
return 0;
}
```
4. 文件复制:可以使用二进制文件流来实现文件复制,将一个文件的内容复制到另一个文件中。
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ifstream source("example.txt", ios::binary);
ofstream dest("copy.txt", ios::binary);
if (source.is_open() && dest.is_open())
{
dest << source.rdbuf();
source.close();
dest.close();
}
else
{
cout << "Unable to open file" << endl;
}
return 0;
}
```
以上是一些常用的文件操作方式,可以根据实际需求选择相应的方式进行操作。
相关推荐
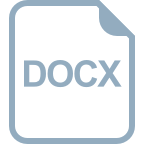
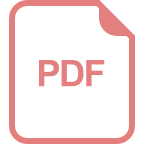













