es6遍历树结构并判断_实现树形结构数据 es6
时间: 2023-12-20 18:07:32 浏览: 98
可以使用ES6中的递归函数来遍历树形结构数据,并判断每个节点的属性是否符合条件。以下是一个示例代码:
```javascript
const treeData = {
name: 'root',
children: [
{
name: 'node1',
children: [
{ name: 'leaf1' },
{ name: 'leaf2' },
],
},
{
name: 'node2',
children: [
{ name: 'leaf3' },
{ name: 'leaf4' },
],
},
],
};
function traverseTree(node, condition) {
if (condition(node)) {
console.log(node.name);
}
if (node.children) {
node.children.forEach(child => traverseTree(child, condition));
}
}
traverseTree(treeData, node => node.name.startsWith('leaf'));
```
这个示例代码遍历了一个树形结构的数据,找到所有节点名称以“leaf”开头的节点,并将其名称输出到控制台。你可以根据实际需求来修改条件判断函数来实现不同的功能。
相关问题
es6 树形结构找到节点
我发现你刚才问过了这个问题,这里是之前的回答:
若要在 ES6 的树形结构中找到一个节点,可以使用递归来实现:
```javascript
function findNode(tree, nodeId) {
if (tree.id === nodeId) {
// 找到节点,返回它
return tree;
} else if (tree.children) {
// 如果当前节点有子节点,遍历子节点
for (let i = 0; i < tree.children.length; i++) {
const result = findNode(tree.children[i], nodeId);
if (result) {
// 找到节点,返回它
return result;
}
}
}
// 没有找到节点,返回 null
return null;
}
// 示例树形结构
const tree = {
id: 1,
children: [
{
id: 2,
children: [
{ id: 4 },
{ id: 5 }
]
},
{
id: 3,
children: [
{ id: 6 },
{ id: 7 }
]
}
]
};
// 找到 id 为 5 的节点
const node = findNode(tree, 5);
console.log(node); // 输出 { id: 5 }
```
在上面的代码中,`findNode` 函数接受两个参数:树形结构和要查找的节点的 ID。如果当前节点的 ID 等于要查找的 ID,就返回当前节点;否则,如果当前节点有子节点,就递归遍历每个子节点,直到找到节点或遍历完所有子节点。如果遍历完所有子节点还没有找到节点,就返回 `null`。
es6 树形结构 循环删除指定id
要在 ES6 的树形结构中删除指定 ID 的节点,可以使用循环和递归相结合的方式来实现:
```javascript
function deleteNode(tree, nodeId) {
// 如果当前节点是要删除的节点,直接返回 null
if (tree.id === nodeId) {
return null;
}
// 如果当前节点有子节点,遍历子节点
if (tree.children) {
for (let i = 0; i < tree.children.length; i++) {
// 递归调用 deleteNode 函数,删除子节点
tree.children[i] = deleteNode(tree.children[i], nodeId);
}
// 过滤掉子节点中值为 null 的节点
tree.children = tree.children.filter(child => child !== null);
}
// 返回当前节点
return tree;
}
// 示例树形结构
const tree = {
id: 1,
children: [
{
id: 2,
children: [
{ id: 4 },
{ id: 5 }
]
},
{
id: 3,
children: [
{ id: 6 },
{ id: 7 }
]
}
]
};
// 删除 id 为 5 的节点
const newTree = deleteNode(tree, 5);
console.log(newTree);
/* 输出
{
id: 1,
children: [
{
id: 2,
children: [
{ id: 4 }
]
},
{
id: 3,
children: [
{ id: 6 },
{ id: 7 }
]
}
]
}
*/
```
在上面的代码中,`deleteNode` 函数接受两个参数:树形结构和要删除的节点的 ID。如果当前节点是要删除的节点,直接返回 `null`,表示这个节点已经被删除。如果当前节点有子节点,就递归调用 `deleteNode` 函数,删除子节点中的指定节点。然后,过滤掉子节点中值为 `null` 的节点,表示这些节点已经被删除。最后,返回当前节点,表示这个节点没有被删除。
阅读全文
相关推荐
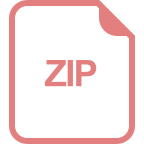
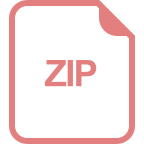
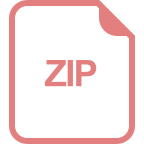
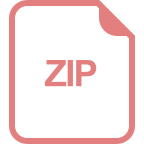
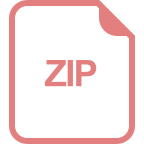
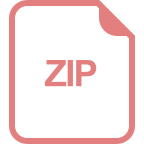
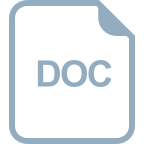
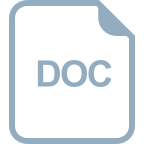
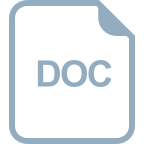
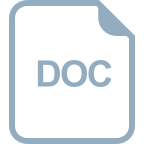
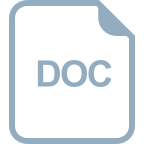
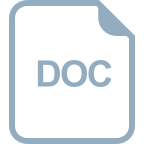
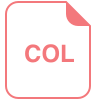
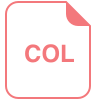