c++ 判断std::vector中元素是否存在
时间: 2023-11-08 19:19:29 浏览: 49
在C++中,我们可以使用`std::find`算法来判断`std::vector`中元素是否存在。`std::find`算法在`<algorithm>`头文件中定义,它接受两个迭代器和一个值作为参数,返回一个迭代器,指向第一个等于该值的元素。如果该值不存在于容器中,则返回指向容器尾部的迭代器。
下面是一个示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main()
{
vector<int> myVec = {1, 2, 3, 4, 5};
if (find(myVec.begin(), myVec.end(), 3) != myVec.end())
{
cout << "Element 3 exists in the vector" << endl;
}
else
{
cout << "Element 3 does not exist in the vector" << endl;
}
if (find(myVec.begin(), myVec.end(), 6) != myVec.end())
{
cout << "Element 6 exists in the vector" << endl;
}
else
{
cout << "Element 6 does not exist in the vector" << endl;
}
return 0;
}
```
输出结果为:
```
Element 3 exists in the vector
Element 6 does not exist in the vector
```
在上面的示例代码中,我们使用`find`算法来判断`myVec`中是否存在值为3和6的元素,输出相应的结果。如果`find`算法返回的迭代器指向容器尾部,则说明该值不存在于容器中。
相关推荐
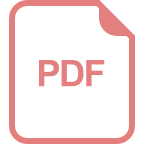
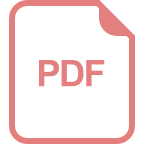














