如何用链表实现笔记管理
时间: 2023-08-15 07:21:49 浏览: 123
使用链表来实现笔记管理,可以实现更加灵活和高效的增删改查操作。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 笔记结构体
struct Note {
char title[50]; // 标题
char content[200]; // 内容
struct Note *next; // 下一个节点指针
};
// 创建新笔记
struct Note *createNote() {
struct Note *note = (struct Note*)malloc(sizeof(struct Note));
printf("请输入标题:");
scanf("%s", note->title);
printf("请输入内容:");
scanf("%s", note->content);
note->next = NULL;
return note;
}
// 添加笔记
void addNote(struct Note **head) {
struct Note *newNote = createNote();
if (*head == NULL) {
*head = newNote;
} else {
struct Note *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = newNote;
}
printf("笔记添加成功!\n");
}
// 编辑笔记
void editNote(struct Note *head) {
if (head == NULL) {
printf("当前没有笔记!\n");
return;
}
char title[50];
printf("请输入要编辑的笔记的标题:");
scanf("%s", title);
struct Note *p = head;
while (p != NULL) {
if (strcmp(p->title, title) == 0) {
printf("请输入新内容:");
scanf("%s", p->content);
printf("笔记编辑成功!\n");
return;
}
p = p->next;
}
printf("未找到该笔记!\n");
}
// 删除笔记
void deleteNote(struct Note **head) {
if (*head == NULL) {
printf("当前没有笔记!\n");
return;
}
char title[50];
printf("请输入要删除的笔记的标题:");
scanf("%s", title);
if (strcmp((*head)->title, title) == 0) {
struct Note *temp = *head;
*head = (*head)->next;
free(temp);
printf("笔记删除成功!\n");
return;
}
struct Note *p = *head;
while (p->next != NULL) {
if (strcmp(p->next->title, title) == 0) {
struct Note *temp = p->next;
p->next = temp->next;
free(temp);
printf("笔记删除成功!\n");
return;
}
p = p->next;
}
printf("未找到该笔记!\n");
}
// 查看笔记
void viewNote(struct Note *head) {
if (head == NULL) {
printf("当前没有笔记!\n");
return;
}
printf("所有笔记如下:\n");
struct Note *p = head;
while (p != NULL) {
printf("标题:%s\n", p->title);
printf("内容:%s\n", p->content);
p = p->next;
}
}
int main() {
struct Note *head = NULL; // 头结点指针初始化为空
int choice;
while (1) {
printf("请选择操作:\n");
printf("1. 添加笔记\n");
printf("2. 编辑笔记\n");
printf("3. 删除笔记\n");
printf("4. 查看笔记\n");
printf("5. 退出\n");
printf("请输入序号:");
scanf("%d", &choice);
switch (choice) {
case 1:
addNote(&head); // 传入头结点指针的地址
break;
case 2:
editNote(head);
break;
case 3:
deleteNote(&head); // 传入头结点指针的地址
break;
case 4:
viewNote(head);
break;
case 5:
printf("退出笔记管理系统!\n");
return 0;
default:
printf("无效的选择!\n");
break;
}
}
}
```
上述代码中,我们使用了链表来存储笔记,每个笔记结构体中包含了标题、内容和指向下一个笔记结构体的指针。在添加、编辑、查看和删除笔记时,我们都使用指针来遍历链表,并实现相应的操作。
需要注意的是,在添加和删除笔记时,需要传入头结点指针的地址,因为头结点指针可能会发生变化,我们需要在函数中修改头结点指针的值。
阅读全文
相关推荐





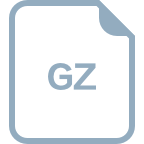












