详细介绍一下 PyTorch
时间: 2023-11-09 18:53:13 浏览: 35
PyTorch是一个基于Python的科学计算包,主要针对两类人群:NumPy使用者和深度学习研究者。它既可以作为NumPy的替代品,又可以提供最大的灵活性和速度,使GPU加速的深度神经网络编程更加容易。同时,它也是一个动态的神经网络工具包,与TensorFlow等静态工具包相比,其编程方式更加直观。
相关问题
介绍一下pytorch的库函数
PyTorch提供了许多功能强大的库函数,用于构建和训练深度学习模型。下面是一些常用的PyTorch库函数的介绍:
1. `torch.Tensor`:PyTorch中的核心数据结构是Tensor,它类似于NumPy的数组,但可以在GPU上加速计算。它提供了各种操作,如数学运算、索引、切片等,可以方便地处理多维数组。
2. `torch.nn`:PyTorch的`torch.nn`模块提供了用于构建神经网络的各种类和函数。它包括了常见的层(如全连接层、卷积层、循环神经网络层等)和激活函数(如ReLU、sigmoid、softmax等),可以通过继承`nn.Module`来自定义网络模型。
3. `torch.optim`:`torch.optim`模块提供了各种优化算法的实现,如随机梯度下降(SGD)、Adam、RMSprop等。它可以用于更新网络模型的参数,并且支持学习率调整等功能。
4. `torch.utils.data`:`torch.utils.data`模块提供了一些工具类,用于处理数据集的加载和预处理。例如,`Dataset`类可以用于创建自定义的数据集,`DataLoader`类可以用于批量加载数据并进行数据增强。
5. `torchvision`:`torchvision`是一个PyTorch的图像处理库,提供了常见的图像数据集(如MNIST、CIFAR-10、ImageNet等)和数据转换工具。它还包含了一些预训练的模型(如ResNet、AlexNet等),可以用于迁移学习或直接使用。
6. `torch.utils.tensorboard`:`torch.utils.tensorboard`模块提供了与TensorBoard集成的功能,可以用于可视化训练过程和模型的性能。它可以记录标量、图像、直方图等信息,并在TensorBoard中展示。
除了上述库函数外,PyTorch还提供了其他许多功能模块,如数据并行处理、模型保存和加载、分布式训练等。这些库函数和功能模块使得PyTorch成为一个完整的深度学习框架,可以满足不同应用场景的需求。
介绍一下PyTorch
PyTorch是一个基于Python的科学计算库,它有强大的GPU加速功能,可以实现高效的深度学习算法。PyTorch的主要特点是动态图机制,使得开发者可以更加灵活地构建神经网络模型。此外,PyTorch还提供了许多优秀的工具包,如torchvision、torchaudio等,方便用户进行图像和音频处理等任务。
相关推荐
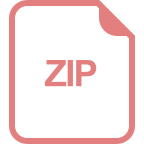
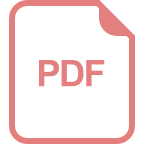
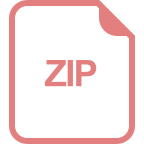













