用pytorch实现一个带注意力机制的图神经网络
时间: 2023-07-20 10:07:25 浏览: 155
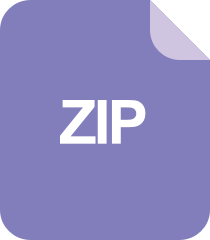
GATE-master_pytorch实现gate_gate_注意力机制_自注意力机制_自编码_

好的,下面是一个简单的带注意力机制的图神经网络的PyTorch实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class GraphAttentionLayer(nn.Module):
def __init__(self, in_features, out_features, dropout, alpha, concat=True):
super(GraphAttentionLayer, self).__init__()
self.in_features = in_features
self.out_features = out_features
self.dropout = dropout
self.alpha = alpha
self.concat = concat
self.W = nn.Parameter(torch.zeros(size=(in_features, out_features)))
nn.init.xavier_uniform_(self.W.data, gain=1.414)
self.a = nn.Parameter(torch.zeros(size=(2*out_features, 1)))
nn.init.xavier_uniform_(self.a.data, gain=1.414)
self.leakyrelu = nn.LeakyReLU(self.alpha)
def forward(self, input, adj):
h = torch.mm(input, self.W)
N = h.size()[0]
a_input = torch.cat([h.repeat(1, N).view(N*N, -1), h.repeat(N, 1)], dim=1).view(N, -1, 2*self.out_features)
e = self.leakyrelu(torch.matmul(a_input, self.a).squeeze(2))
zero_vec = -9e15*torch.ones_like(e)
attention = torch.where(adj > 0, e, zero_vec)
attention = F.softmax(attention, dim=1)
attention = F.dropout(attention, self.dropout, training=self.training)
h_prime = torch.matmul(attention, h)
if self.concat:
return F.elu(h_prime)
else:
return h_prime
```
这个代码实现了一个带注意力机制的图神经网络的层,其中包含输入特征的维度in_features,输出特征的维度out_features,dropout率dropout,LeakyReLU激活函数的负斜率alpha,以及一个可选的参数concat,用于指定是否将输入和输出连接在一起。
这个层接受一个输入特征张量input和一个邻接矩阵adj,计算出带注意力机制的输出特征张量。具体来说,它首先将输入特征张量input与权重矩阵W相乘,得到一个中间特征张量h。然后,它将中间特征张量h复制N次,并将每个副本与其他副本拼接在一起,得到一个形状为(N, N, 2*out_features)的张量a_input。张量a_input将作为参数矩阵a的输入,通过矩阵乘法和LeakyReLU激活函数得到一个张量e。该层根据邻接矩阵adj和张量e计算注意力张量attention,对其进行dropout,并将其与中间特征张量h相乘得到带注意力机制的输出特征张量h_prime。如果concat参数为True,则将输入特征张量input和输出特征张量h_prime沿着特征维度拼接在一起,否则只返回输出特征张量h_prime。
注意,这个实现假设输入特征张量input的第一维是节点数N。如果你的数据不是这种形式,你需要对代码进行修改。
阅读全文
相关推荐















