用pytorch对一张图片的注意力图可视化
时间: 2023-07-16 20:12:18 浏览: 51
要对一张图片的注意力图进行可视化,需要使用卷积神经网络中的注意力机制,比如是self-attention或者是multi-head attention。
以下是一个基于pytorch的示例代码,可以对一张图片进行可视化:
```python
import torch
import torch.nn.functional as F
import matplotlib.pyplot as plt
from torchvision import transforms
from PIL import Image
# 加载图片并进行预处理
image = Image.open('test.jpg')
preprocess = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
])
image_tensor = preprocess(image).unsqueeze(0) # 增加batch维度
# 加载模型,并使用self-attention
model = torch.hub.load('pytorch/vision', 'resnet50', pretrained=True)
model.eval()
modules = list(model.children())
attention_module = modules[-2] # 取ResNet50的最后一层
for i, module in enumerate(attention_module.children()):
if isinstance(module, torch.nn.MultiheadAttention):
attention_layer = module
break
# 获取注意力值
output, attention = attention_layer(image_tensor, image_tensor, image_tensor)
attention = F.softmax(attention, dim=-1) # 归一化
# 绘制注意力图
attention = attention.squeeze(0).detach().cpu()
attention = transforms.Resize((224, 224))(attention)
plt.imshow(attention, cmap='gray')
plt.axis('off')
plt.show()
```
在上述代码中,我们首先加载了一张图片并进行了预处理。然后,我们加载了ResNet50模型,并使用最后一层的self-attention。接着,我们将图片输入到模型中,得到输出和注意力值。最后,我们将注意力值进行归一化并绘制出来。
需要注意的是,由于注意力图是在模型中生成的,因此不同的模型可能会有不同的实现方式,所以需要根据实际情况进行修改。
相关推荐
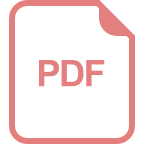














