已知存在个数为 n 的整数数组序列 in _ data [ n ],按照从高到低的顺序对数组进行排序,并根据不同情况计算中值,用C语言实现
时间: 2024-09-13 12:03:53 浏览: 37
在C语言中,我们可以使用标准库函数`qsort()`来进行数组的降序排序,然后找到中位数。如果n是奇数,中值就是排序后的数组中间元素;如果是偶数,中值则是中间两个元素的平均值。以下是简单的步骤和代码示例:
首先,我们需要编写一个比较函数,用于`qsort()`,它会按照元素值的大小进行降序排列:
```c
int compare(const void* a, const void* b) {
return (*(const int*)b - *(const int*)a);
}
```
接下来,我们创建主函数,包含排序和计算中值的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 中值计算函数
double calculate_median(int arr[], int n) {
// 先按降序排列数组
qsort(arr, n, sizeof(int), compare);
// 计算并返回中值
if (n % 2 == 0) { // 如果数组长度是偶数
int mid = n / 2;
return (arr[mid - 1] + arr[mid]) / 2.0; // 平均中间两个数
} else { // 如果数组长度是奇数
return arr[n / 2]; // 只有一个中间数
}
}
int main() {
int in_data[] = {5, 2, 9, 1, 7, 6}; // 你的输入数组
int n = sizeof(in_data) / sizeof(in_data[0]);
printf("Sorted array: ");
for (int i = 0; i < n; i++) {
printf("%d ", in_data[i]);
}
double median = calculate_median(in_data, n);
printf("\nMedian is: %.2f\n", median);
return 0;
}
```
阅读全文
相关推荐
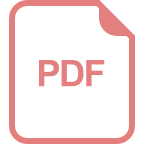
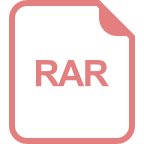
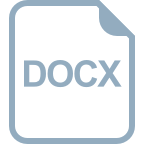

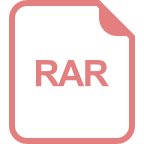
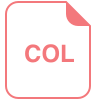
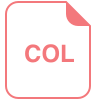
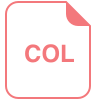
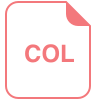
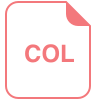
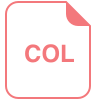
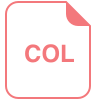
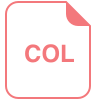
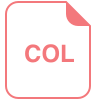
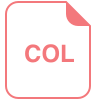
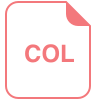
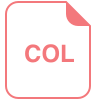


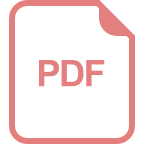