帮我用java写一个文件上传的方法
时间: 2023-01-11 10:55:36 浏览: 81
当然,我很乐意帮助你。
你可以使用 Java 的 `HttpURLConnection` 类来上传文件。下面是一个简单的例子:
```
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileUpload {
public static void main(String[] args) {
String fileName = "example.txt";
String urlString = "http://www.example.com/upload";
try {
// 打开连接
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求属性
connection.setRequestProperty("Content-Type", "multipart/form-data");
connection.setRequestProperty("Content-Disposition", "form-data; name=\"file\"; filename=\"" + fileName + "\"");
connection.setRequestMethod("POST");
connection.setDoOutput(true);
// 写入文件内容
OutputStream outputStream = connection.getOutputStream();
FileInputStream fileInputStream = new FileInputStream(fileName);
byte[] buffer = new byte[1024];
int length;
while ((length = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
fileInputStream.close();
outputStream.flush();
outputStream.close();
// 读取响应
int responseCode = connection.getResponseCode();
InputStream inputStream;
if (responseCode == HttpURLConnection.HTTP_OK) {
inputStream = connection.getInputStream();
} else {
inputStream = connection.getErrorStream();
}
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// 断开连接
connection.disconnect();
// 打印响应
System.out.println(response.toString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这段代码中,我们使用了 `HttpURLConnection` 类来打开一个连接,设置请求属性,并写入文件内容。然后,我们读取服务器的响应,并打印出来。最后,我
相关推荐
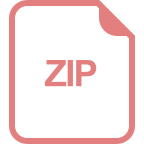
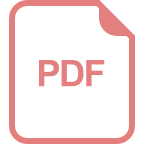














