<el-table-column v-model="infoDataList.is_selected" type="selection" width="55"> </el-table-column>0
时间: 2024-05-30 20:10:25 浏览: 74
这是一个基于 Element UI 的表格组件中的列定义,其中:
- `v-model="infoDataList.is_selected"` 表示该列数据的选中状态会与 `infoDataList.is_selected` 双向绑定,即在表格中选中/取消选中某行数据时,该属性值会自动更新。
- `type="selection"` 表示该列是用来展示行数据的选中状态的。
- `width="55"` 表示该列的宽度为 55 像素。
相关问题
vue el-table type="selection" 回显
在Vue.js中,`el-table`组件的`type="selection"`属性通常用于创建一个可以选中的表格,让用户能够勾选某一行或多行数据。如果你想要实现回显功能,即表格的数据已经预先存在并且需要在页面加载时显示已选择的状态,你需要做以下几个步骤:
1. **绑定数据**: 首先,确保你有一个包含数据的数组,并且每个数据项都有一个标识状态的字段,比如`selected`布尔值。
```js
data() {
return {
tableData: [
{ id: 1, name: 'A', selected: false },
{ id: 2, name: 'B', selected: true },
// ...
],
};
}
```
2. **配置表头**: 在`el-table-column`的模板里,包含一个复选框列,并设置其默认值等于`selected`字段。
```html
<template>
<el-table :data="tableData">
<el-table-column type="selection" width="55"></el-table-column>
<el-table-column prop="name" label="Name"></el-table-column>
<!-- ...其他列 -->
<el-table-column label="Selected">
<template slot-scope="{ $index, row }">
<el-checkbox v-model="row.selected" @change="handleCheckboxChange(row)"></el-checkbox>
</template>
</el-table-column>
</el-table>
</template>
```
3. **处理事件**: 创建一个方法来监听复选框变化,当用户改变某个条目的选择状态时更新状态。
```js
methods: {
handleCheckboxChange(row) {
// 更新数据数组中对应行的selected值
this.tableData.find(item => item.id === row.id).selected = row.selected;
}
},
```
4. **初始化回显**: 当表格组件挂载时,遍历数据并根据`selected`字段预设复选框的状态。
```js
mounted() {
this.tableData.forEach(row => (this.$refs.multipleTable.selection.rows[row.id] = row.selected));
}
```
这样就实现了`el-table`的回显功能,数据加载完成后会根据`selected`字段自动勾选相应的行。
如何向el-table-column中添加el-radio
根据提供的引用内容,可以向el-table-column中添加el-radio来实现单选效果。以下是一个示例代码:
```html
<template>
<el-table :data="tableData" style="width: 100%">
<el-table-column type="selection"></el-table-column>
<el-table-column label="姓名" prop="name"></el-table-column>
<el-table-column label="年龄" prop="age"></el-table-column>
<el-table-column label="性别">
<template slot-scope="scope">
<el-radio v-model="selected" :label="scope.row.id"></el-radio>
</template>
</el-table-column>
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, name: '张三', age: 20 },
{ id: 2, name: '李四', age: 25 },
{ id: 3, name: '王五', age: 30 }
],
selected: null
};
}
};
</script>
```
在上述代码中,我们使用了el-table-column的type属性来实现单选效果。通过在el-table-column中使用template标签,并使用slot-scope来获取每一行的数据,然后使用el-radio来实现单选效果。v-model绑定的是selected变量,通过设置label属性为每一行的唯一标识符,来实现单选效果。
阅读全文
相关推荐
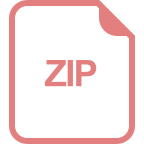
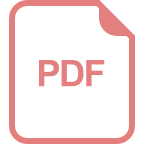
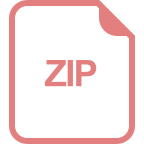













