c语言有头单链表怎么变成无头单链表举例
时间: 2023-11-25 18:07:09 浏览: 82
将头节点删除,使第二个节点成为新的头节点即可将头单链表变成无头单链表。
举例:
假设有以下头单链表:
```
head -> 1 -> 2 -> 3 -> 4 -> NULL
```
要将其变成无头单链表,可以先定义一个指针指向第二个节点:
```
Node *newHead = head->next;
```
然后将头节点删除:
```
free(head);
```
最后,将新的头节点设置为链表的第一个节点:
```
head = newHead;
```
这样就将头单链表转变成了无头单链表:
```
1 -> 2 -> 3 -> 4 -> NULL
```
相关问题
c语言表示出单链表
单链表可以用C语言实现,以下是一个简单的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 创建节点
struct ListNode* createNode(int val) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
return newNode;
}
// 插入节点
void insertNode(struct ListNode** head, int val) {
struct ListNode* newNode = createNode(val);
if (*head == NULL) {
*head = newNode;
} else {
struct ListNode* cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
}
// 删除节点
void deleteNode(struct ListNode** head, int val) {
if (*head == NULL) {
return;
}
struct ListNode* cur = *head;
struct ListNode* prev = NULL;
while (cur != NULL && cur->val != val) {
prev = cur;
cur = cur->next;
}
if (cur == NULL) {
return;
}
if (prev == NULL) {
*head = cur->next;
} else {
prev->next = cur->next;
}
free(cur);
}
// 打印链表
void printList(struct ListNode* head) {
struct ListNode* cur = head;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
struct ListNode* head = NULL;
insertNode(&head, 1);
insertNode(&head, 2);
insertNode(&head, 3);
insertNode(&head, 4);
printList(head);
deleteNode(&head, 2);
printList(head);
return 0;
}
```
这个示例代码实现了单链表的基本操作,包括创建节点、插入节点、删除节点和打印链表。你可以根据你的需求进行修改。
c语言如何创建单链表
在C语言中创建单链表通常需要以下几个步骤:
1. 定义节点结构体(Node):
```c
typedef struct Node {
int data; // 节点的数据域
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. 创建链表头结点(List初始化):
```c
Node* head = NULL; // 初始化链表为空
```
3. 插入元素到链表:
- 插入在头部:`head = (Node*)malloc(sizeof(Node)); // 分配内存`
```c
head->data = value;
head->next = previous_head;
previous_head = head;
```
- 插入在指定位置:找到前一个节点并更新 `next` 指针。
4. 删除元素(如果需要):
- 需要知道要删除节点的值或下一个节点的指针
```c
Node* temp = head;
while (temp != NULL && temp->data != target_value) {
temp = temp->next;
}
if (temp != NULL) {
Node* to_delete = temp;
temp = temp->next;
free(to_delete);
if (temp == NULL) { // 如果刚删除的是最后一个节点
head = NULL;
} else {
to_delete->next = temp;
}
}
```
5. 遍历链表:
```c
Node* current = head;
while (current != NULL) {
printf("%d ", current->data); // 打印数据
current = current->next;
}
```
阅读全文
相关推荐
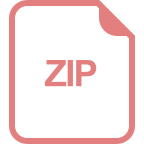
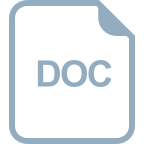
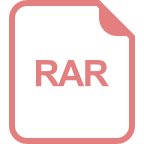
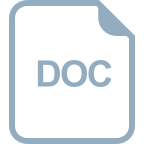



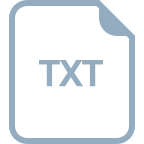
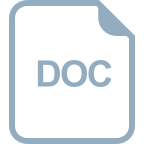


