管道创建和互斥读写的系统函数的调用方法
时间: 2024-04-29 15:25:26 浏览: 12
管道创建函数:pipe()
函数原型:int pipe(int fd[2]);
函数说明:创建一个管道,fd数组中fd[0]表示管道读端,fd[1]表示管道写端。
示例代码:
```c
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
int fd[2];
char buf[1024];
pid_t pid;
if (pipe(fd) < 0) {
perror("pipe");
exit(1);
}
if ((pid = fork()) < 0) {
perror("fork");
exit(1);
} else if (pid == 0) { // 子进程
close(fd[0]);
char* msg = "hello, parent!";
write(fd[1], msg, strlen(msg));
close(fd[1]);
exit(0);
} else { // 父进程
close(fd[1]);
int n;
if ((n = read(fd[0], buf, sizeof(buf))) < 0) {
perror("read");
exit(1);
}
printf("parent received: %s\n", buf);
close(fd[0]);
exit(0);
}
}
```
互斥读写的系统函数:pthread_mutex_init()、pthread_mutex_lock()、pthread_mutex_unlock()
函数原型:
```c
int pthread_mutex_init(pthread_mutex_t* mutex, const pthread_mutexattr_t* attr);
int pthread_mutex_lock(pthread_mutex_t* mutex);
int pthread_mutex_unlock(pthread_mutex_t* mutex);
```
函数说明:
- pthread_mutex_init:初始化互斥锁。
- pthread_mutex_lock:加锁。
- pthread_mutex_unlock:解锁。
示例代码:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int sum = 0;
void* thread_func(void* arg) {
int i;
for (i = 0; i < 1000000; i++) {
pthread_mutex_lock(&mutex);
sum += 1;
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t tid1, tid2;
if (pthread_create(&tid1, NULL, thread_func, NULL) != 0) {
perror("pthread_create");
exit(1);
}
if (pthread_create(&tid2, NULL, thread_func, NULL) != 0) {
perror("pthread_create");
exit(1);
}
if (pthread_join(tid1, NULL) != 0) {
perror("pthread_join");
exit(1);
}
if (pthread_join(tid2, NULL) != 0) {
perror("pthread_join");
exit(1);
}
printf("sum: %d\n", sum);
return 0;
}
```
相关推荐
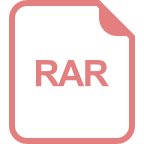
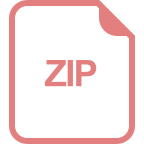
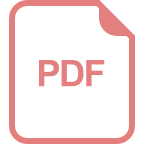














