int SetUpTCPtoSendInformation(char option[], char *command) { static char *TCPCommand; if (strstr(option, "set")) { TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { CON_LOG("Failed to set and obtain TCP command variable memory allocation"); return 0; } strcpy(TCPCommand, command); return 1; } else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand) ) { strcpy(command, TCPCommand); memset(TCPCommand, '\0', strlen(TCPCommand)); isTCPCommandSet = 0; free(TCPCommand); return 1; } strcpy(command, ""); return 0; } char a[32] = "zhangsan"; SetUpTCPtoSendInformation("set","zhangsan"); SetUpTCPtoSendInformation("get",a); printf("%s",a); SetUpTCPtoSendInformation("get",a); printf("%s",a);
时间: 2024-04-09 22:32:19 浏览: 47
上述代码是一个用于设置和获取TCP命令的函数。首先,函数接受一个选项参数和一个命令参数。如果选项参数是"set",则将命令参数分配给TCPCommand变量,并返回1表示设置成功。如果选项参数是"get",并且TCPCommand变量不为空且长度不为零,则将TCPCommand的值复制给命令参数,并将TCPCommand重置为NULL,并释放TCPCommand的内存,最后返回1表示获取成功。如果以上条件都不满足,则将命令参数置空,并返回0表示设置或获取失败。
接下来,代码声明了一个字符数组a,并将其初始化为"zhangsan"。然后通过调用SetUpTCPtoSendInformation函数进行设置操作,传入选项参数"set"和命令参数"a"。再次调用SetUpTCPtoSendInformation函数进行获取操作,将获取到的值赋给数组a,并打印出来。最后再次调用SetUpTCPtoSendInformation函数进行获取操作,并将获取到的值赋给数组a,并打印出来。
根据代码逻辑,最终打印的结果应该是两次获取到的值,即"zhangsan"。
相关问题
int SetUpTCPtoSendInformation(char option[],char * command) { static char * TCPCommand; if(strstr(option,"set")) { TCPCommand=malloc(strlen(command) + 1); strcpy(TCPCommand,command); } else if(strstr(option,"get")) { strcpy(command,TCPCommand); free(TCPCommand); } } char a[32] = "zhngsan"; SetUpTCPtoSendInformation("set",a); SetUpTCPtoSendInformation("get",a); printf("%s",a);
根据你提供的代码,`SetUpTCPtoSendInformation` 函数用于设置和获取 TCP 命令。你通过传递不同的选项和命令来执行不同的操作。
在这个例子中,你首先将字符串 "zhngsan" 赋值给字符数组 `a`。然后,你调用 `SetUpTCPtoSendInformation` 函数两次,一次传递 "set" 选项和 `a` 数组作为命令,另一次传递 "get" 选项和 `a` 数组作为命令。
根据函数的逻辑,当选项为 "set" 时,它会动态分配内存并将命令复制到 `TCPCommand` 变量中。当选项为 "get" 时,它会将 `TCPCommand` 变量中的值复制到命令数组中,并释放动态分配的内存。
在 `printf` 语句中,你打印了 `a` 数组的值。
根据这段代码的执行顺序,最终 `a` 数组的值将为 "zhngsan"。原因是第二次调用 `SetUpTCPtoSendInformation` 函数时,选项为 "get",它会将 `TCPCommand` 变量中的值复制到命令数组 `a` 中。由于在第一次调用时没有更改 `TCPCommand` 的值,所以它仍然指向 "zhngsan"。
因此,最终打印的结果将是 "zhngsan"。
请注意,你的 `SetUpTCPtoSendInformation` 函数没有返回值,在这种情况下,最好将其声明为 `void` 类型。同时,为了避免内存泄漏,你可能需要在函数中处理一些异常情况,例如 `malloc` 失败时需要释放已分配的内存。
int SetUpTCPtoSendInformation(char option[], char *command) { static char *TCPCommand; if (strstr(option, "set")) { TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { CON_LOG("Failed to set and obtain TCP command variable memory allocation"); return 0; } strcpy(TCPCommand, command); return 1; } else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) { strcpy(command, TCPCommand); memset(TCPCommand, '\0', strlen(TCPCommand)); free(TCPCommand); return 1; } memset(command,0,sizeof(command)); strcpy(command, ""); return 0; } 上面使用锁避免多个进程同时访问
在上面的代码中,并没有使用锁来避免多个进程同时访问。你可以使用互斥锁(mutex)来实现对共享资源的同步访问。下面是一个使用互斥锁的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
static char *TCPCommand;
static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int SetUpTCPtoSendInformation(char option[], char *command) {
pthread_mutex_lock(&mutex); // 加锁
if (strstr(option, "set")) {
TCPCommand = malloc(strlen(command) + 1);
if (TCPCommand == NULL) {
CON_LOG("Failed to set and obtain TCP command variable memory allocation");
pthread_mutex_unlock(&mutex); // 解锁
return 0;
}
strcpy(TCPCommand, command);
pthread_mutex_unlock(&mutex); // 解锁
return 1;
} else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) {
strcpy(command, TCPCommand);
memset(TCPCommand, '\0', strlen(TCPCommand));
free(TCPCommand);
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
memset(command, 0, sizeof(command));
strcpy(command, "");
pthread_mutex_unlock(&mutex); // 解锁
return 0;
}
```
在代码中,我们使用了 `pthread_mutex_t` 类型的互斥锁,并使用 `pthread_mutex_lock` 和 `pthread_mutex_unlock` 函数来分别加锁和解锁。这样可以确保在访问共享资源(`TCPCommand`)时只有一个线程可以进行操作,避免了多个进程同时访问的问题。
阅读全文
相关推荐
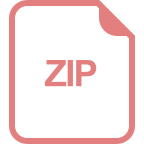
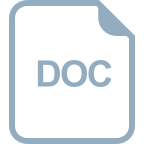
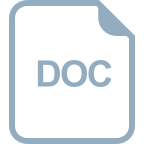








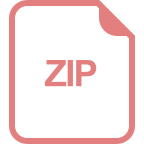
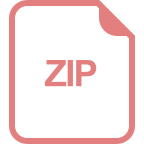