python实现蚁群算法
时间: 2023-10-20 15:05:56 浏览: 45
蚁群算法是一个基于模拟蚂蚁在寻找食物过程中的行为而发展的一种优化算法。下面是使用python实现蚁群算法的代码示例:
```python
import random
# 蚁群算法参数设置
alpha = 1 # 信息素重要程度因子
beta = 2 # 启发函数重要程度因子
rho = 0.5 # 信息素挥发速度
Q = 100 # 信息素增加强度因子
ant_count = 10 # 蚂蚁数量
generations = 50 # 迭代次数
# 城市距离矩阵,也可以从外部文件读取
distance = [
[0, 1, 2, 3, 4],
[1, 0, 1, 2, 3],
[2, 1, 0, 1, 2],
[3, 2, 1, 0, 1],
[4, 3, 2, 1, 0]
]
# 初始化信息素矩阵
pheromone = [[1.0 for j in range(5)] for i in range(5)]
# 计算路径长度
def path_length(path):
length = 0
for i in range(1, len(path)):
length += distance[path[i - 1]][path[i]]
return length
# 选择下一个城市
def select_next_city(current_city, allowed_cities):
# 计算各个城市的概率
probabilities = []
total = 0
for city in allowed_cities:
# 计算信息素和启发函数的乘积
p = pow(pheromone[current_city][city], alpha) * pow(1.0 / distance[current_city][city], beta)
probabilities.append(p)
total += p
# 根据概率选择下一个城市
r = random.uniform(0, total)
acc = 0
for i in range(len(allowed_cities)):
acc += probabilities[i]
if acc >= r:
return allowed_cities[i]
# 如果没有合适的城市,则随机选择一个
return allowed_cities[random.randint(0, len(allowed_cities) - 1)]
# 更新信息素矩阵
def update_pheromone(ants):
# 信息素挥发
for i in range(len(pheromone)):
for j in range(len(pheromone[i])):
pheromone[i][j] *= (1 - rho)
# 信息素增加
for ant in ants:
length = path_length(ant)
for i in range(1, len(ant)):
pheromone[ant[i - 1]][ant[i]] += Q / length
pheromone[ant[-1]][ant[0]] += Q / length
# 蚁群算法主程序
def ant_colony():
best_path = None
best_length = float('inf')
for g in range(generations):
# 初始化蚂蚁
ants = [[] for i in range(ant_count)]
for i in range(ant_count):
ants[i].append(random.randint(0, len(distance) - 1))
# 让蚂蚁移动
for i in range(len(ants[0]) - 1, len(distance)):
for ant in ants:
allowed_cities = list(set(range(len(distance))) - set(ant))
next_city = select_next_city(ant[-1], allowed_cities)
ant.append(next_city)
# 更新最佳路径
for ant in ants:
length = path_length(ant)
if length < best_length:
best_length = length
best_path = ant
# 更新信息素矩阵
update_pheromone(ants)
return best_path, best_length
# 测试
best_path, best_length = ant_colony()
print('Best Path:', best_path, 'Best Length:', best_length)
```
在上面的代码中,我们首先定义了蚁群算法的参数,包括信息素重要程度因子、启发函数重要程度因子、信息素挥发速度、信息素增加强度因子、蚂蚁数量、迭代次数等。然后,我们初始化了城市距离矩阵和信息素矩阵。
在主程序中,我们首先初始化了蚂蚁,并让它们移动,直到所有城市都被访问过。在移动过程中,我们使用了选择下一个城市和更新信息素矩阵两个函数。在每次迭代结束后,我们更新了最佳路径和最短路径长度,并更新了信息素矩阵。
最后,我们运行蚁群算法,并输出最佳路径和最短路径长度。
相关推荐
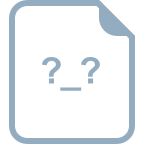
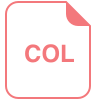

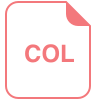
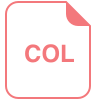
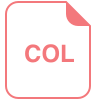









