霍夫变换检测直线找到并画出其交点c++
时间: 2023-08-11 14:27:20 浏览: 273
以下是基于OpenCV的C++代码,可以检测图像中的直线,并找到交点:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <cmath>
using namespace cv;
using namespace std;
int main()
{
// 读入图片
Mat img = imread("image.jpg");
// 灰度化
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
// 边缘检测
Mat edges;
Canny(gray, edges, 50, 150, 3);
// 霍夫变换
vector<Vec2f> lines;
HoughLines(edges, lines, 1, CV_PI / 180, 200);
// 画出直线
for (size_t i = 0; i < lines.size(); i++)
{
float rho = lines[i][0];
float theta = lines[i][1];
Point pt1, pt2;
double a = cos(theta), b = sin(theta);
double x0 = a * rho, y0 = b * rho;
pt1.x = cvRound(x0 + 1000 * (-b));
pt1.y = cvRound(y0 + 1000 * a);
pt2.x = cvRound(x0 - 1000 * (-b));
pt2.y = cvRound(y0 - 1000 * a);
line(img, pt1, pt2, Scalar(0, 0, 255), 3, LINE_AA);
}
// 计算交点
vector<Point2f> points;
for (size_t i = 0; i < lines.size(); i++)
{
for (size_t j = i + 1; j < lines.size(); j++)
{
float rho1 = lines[i][0], theta1 = lines[i][1];
float rho2 = lines[j][0], theta2 = lines[j][1];
Mat A = (Mat_<float>(2, 2) << cos(theta1), sin(theta1), cos(theta2), sin(theta2));
Mat b = (Mat_<float>(2, 1) << rho1, rho2);
Mat x;
solve(A, b, x, DECOMP_LU);
Point2f pt(x.at<float>(0, 0), x.at<float>(1, 0));
points.push_back(pt);
}
}
// 画出交点
for (size_t i = 0; i < points.size(); i++)
{
circle(img, points[i], 5, Scalar(0, 255, 0), -1);
}
// 显示结果
imshow("result", img);
waitKey(0);
destroyAllWindows();
return 0;
}
```
与Python代码类似,使用 `HoughLines` 函数进行霍夫变换,得到直线的参数。使用 `line` 函数画出直线。计算交点使用了 OpenCV 的线性方程求解函数 `solve`。最后,使用 `circle` 函数画出交点。
阅读全文
相关推荐

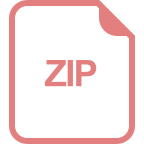
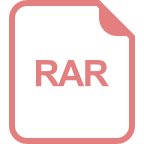












