Only 8-bit grayscale or color images are supported怎么解决?
时间: 2024-01-27 16:03:21 浏览: 142
这个错误通常是由于图像的格式不被支持所导致的。你可以尝试将图像转换为支持的格式,例如使用Pillow库中的`convert`函数来将图像转换为8位灰度或彩色图像。以下是一个例子:
```python
from PIL import Image
# 打开图像
img = Image.open('image.png')
# 将图像转换为8位灰度或彩色图像
img = img.convert('L') # 转换为8位灰度图像
# 或者
img = img.convert('RGB') # 转换为24位彩色图像
# 处理图像
...
# 保存图像
img.save('processed_image.png')
```
如果你的图像格式本身就支持8位灰度或彩色,那么可能需要检查一下你的代码是否正确读取了图像。
相关问题
Only 8-bit grayscale or color images are supported:
This error message indicates that the system or software you are using only supports 8-bit grayscale or color images. This means that images with higher color depths, such as 16-bit or 32-bit color, may not be compatible with the system or software. To resolve this issue, you may need to convert your images to the supported color depth or find a different system or software that can handle higher color depths.
基于pytorch的输入为1111(8-bit color)灰度图像文件夹,用dcgan扩充此数据集,并生成更多单张1111(8-bit color)灰度图像的代码
好的,我可以为您提供一个基于PyTorch和DCGAN的代码示例,用于扩充8位灰度图像数据集并生成更多的单张8位灰度图像。以下是示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
from tqdm import tqdm
# 定义生成器和判别器网络
class Generator(nn.Module):
def __init__(self, nz=100, ngf=64, nc=1):
super(Generator, self).__init__()
self.main = nn.Sequential(
nn.ConvTranspose2d(nz, ngf * 8, 4, 1, 0, bias=False),
nn.BatchNorm2d(ngf * 8),
nn.ReLU(True),
nn.ConvTranspose2d(ngf * 8, ngf * 4, 4, 2, 1, bias=False),
nn.BatchNorm2d(ngf * 4),
nn.ReLU(True),
nn.ConvTranspose2d(ngf * 4, ngf * 2, 4, 2, 1, bias=False),
nn.BatchNorm2d(ngf * 2),
nn.ReLU(True),
nn.ConvTranspose2d(ngf * 2, ngf, 4, 2, 1, bias=False),
nn.BatchNorm2d(ngf),
nn.ReLU(True),
nn.ConvTranspose2d(ngf, nc, 4, 2, 1, bias=False),
nn.Tanh()
)
def forward(self, input):
return self.main(input)
class Discriminator(nn.Module):
def __init__(self, ndf=64, nc=1):
super(Discriminator, self).__init__()
self.main = nn.Sequential(
nn.Conv2d(nc, ndf, 4, 2, 1, bias=False),
nn.LeakyReLU(0.2, inplace=True),
nn.Conv2d(ndf, ndf * 2, 4, 2, 1, bias=False),
nn.BatchNorm2d(ndf * 2),
nn.LeakyReLU(0.2, inplace=True),
nn.Conv2d(ndf * 2, ndf * 4, 4, 2, 1, bias=False),
nn.BatchNorm2d(ndf * 4),
nn.LeakyReLU(0.2, inplace=True),
nn.Conv2d(ndf * 4, ndf * 8, 4, 2, 1, bias=False),
nn.BatchNorm2d(ndf * 8),
nn.LeakyReLU(0.2, inplace=True),
nn.Conv2d(ndf * 8, 1, 4, 1, 0, bias=False),
nn.Sigmoid()
)
def forward(self, input):
return self.main(input)
# 初始化网络和优化器
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
nz = 100 # 噪声向量的大小
ngf = 64 # 生成器的特征图数量
ndf = 64 # 判别器的特征图数量
nc = 1 # 输入图像的通道数
generator = Generator(nz, ngf, nc).to(device)
discriminator = Discriminator(ndf, nc).to(device)
lr = 0.0002 # 学习率
beta1 = 0.5 # Adam优化器的参数
batch_size = 64 # 每个批次的大小
optimizerG = optim.Adam(generator.parameters(), lr=lr, betas=(beta1, 0.999))
optimizerD = optim.Adam(discriminator.parameters(), lr=lr, betas=(beta1, 0.999))
# 加载数据集
transform = transforms.Compose([
transforms.Grayscale(num_output_channels=1),
transforms.Resize(64),
transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))
])
dataset = datasets.ImageFolder('path/to/dataset', transform=transform)
dataloader = DataLoader(dataset, batch_size=batch_size, shuffle=True)
# 定义损失函数和噪声向量
criterion = nn.BCELoss()
fixed_noise = torch.randn(64, nz, 1, 1, device=device)
# 训练网络
num_epochs = 50 # 训练轮数
for epoch in range(num_epochs):
for i, data in tqdm(enumerate(dataloader), total=len(dataloader)):
real_data = data[0].to(device)
batch_size = real_data.size(0)
label_real = torch.full((batch_size,), 1, device=device)
label_fake = torch.full((batch_size,), 0, device=device)
# 训练判别器
discriminator.zero_grad()
output = discriminator(real_data)
loss_D_real = criterion(output, label_real)
loss_D_real.backward()
noise = torch.randn(batch_size, nz, 1, 1, device=device)
fake_data = generator(noise)
output = discriminator(fake_data.detach())
loss_D_fake = criterion(output, label_fake)
loss_D_fake.backward()
loss_D = loss_D_real + loss_D_fake
optimizerD.step()
# 训练生成器
generator.zero_grad()
output = discriminator(fake_data)
loss_G = criterion(output, label_real)
loss_G.backward()
optimizerG.step()
# 输出训练结果
print('[%d/%d] Loss_D: %.4f Loss_G: %.4f' % (epoch + 1, num_epochs, loss_D.item(), loss_G.item()))
# 保存生成的图像
with torch.no_grad():
fake = generator(fixed_noise).detach().cpu()
save_image(fake, 'fake_images_%03d.png' % (epoch + 1), normalize=True)
```
这个代码示例实现了一个简单的DCGAN网络,用于扩充8位灰度图像数据集并生成更多的单张8位灰度图像。您需要将 `path/to/dataset` 替换为您自己的数据集路径,并确保数据集中的所有图像都是8位灰度图像。您还可以根据需要调整超参数,如批次大小,学习率等。在训练过程中,您可以观察到判别器和生成器的损失,并保存生成的图像以进行可视化。
阅读全文
相关推荐
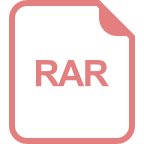
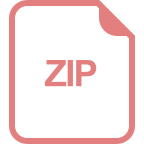
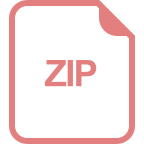













