typescript数组map
时间: 2024-01-23 14:02:48 浏览: 28
TypeScript中的数组map()方法是用于将数组中的每个元素应用于给定的函数,并返回一个新数组,其中包含每个函数调用的结果。该方法不会更改原始数组。下面是一个示例:
```
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(num => num * 2);
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
```
在上面的示例中,我们首先定义了一个名为numbers的数组,然后使用map()方法将每个元素乘以2,并将结果存储在名为doubledNumbers的新数组中。最后,我们使用console.log()方法打印出新数组。
相关问题
ts 数组map方法
在 TypeScript 中,数组的 `map` 方法用于对数组中的每个元素执行指定的操作,并返回一个新的数组,该数组包含操作后的结果。 `map` 方法接收一个回调函数作为参数,该回调函数会被应用于数组中的每个元素。
下面是使用 `map` 方法的示例:
```typescript
let numbers: number[] = [1, 2, 3, 4, 5];
let squaredNumbers = numbers.map((num) => num * num);
console.log(squaredNumbers); // 输出 [1, 4, 9, 16, 25]
```
在上面的示例中,`map` 方法遍历了 `numbers` 数组中的每个元素,并将每个元素平方后返回一个新的数组 `squaredNumbers`。
你还可以在回调函数中执行更复杂的操作。例如,将字符串数组转换为大写形式:
```typescript
let fruits: string[] = ["apple", "banana", "cherry"];
let uppercasedFruits = fruits.map((fruit) => fruit.toUpperCase());
console.log(uppercasedFruits); // 输出 ["APPLE", "BANANA", "CHERRY"]
```
希望这可以帮助到你!如果有任何其他问题,请随时提问。
TypeScript 取数组长度
在 TypeScript 中,可以使用 `.length` 属性获取数组的长度。这个属性返回一个数字,表示数组中元素的数量。
以下是一个示例代码:
```typescript
const arr = [1, 2, 3, 4, 5];
console.log(arr.length); // 输出 5
```
在上面的示例中,我们首先定义了一个包含五个数字的数组。然后,我们使用 `.length` 属性获取数组的长度,并将其打印到控制台中。
请注意,`.length` 属性可以用于任何类型的数组,包括字符串数组、对象数组等。
另外,需要注意的是,对于类似于对象的其他类型,如 Map 和 Set,它们也有一个 `.size` 属性,用于获取它们元素的数量。
相关推荐
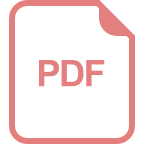
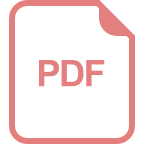












