C#将shp保存txt
时间: 2023-07-27 18:25:56 浏览: 172
你可以使用 C# 中的 OGR 库来读取和处理 Shapefile 文件,并将其保存为文本文件。以下是一个简单的示例代码:
```csharp
using OSGeo.OGR;
// 打开 Shapefile 文件
DataSource ds = Ogr.Open("path/to/shapefile.shp", 0);
// 获取第一个图层
Layer layer = ds.GetLayerByIndex(0);
// 创建文本文件并打开
System.IO.StreamWriter file = new System.IO.StreamWriter("path/to/output.txt");
// 遍历所有要素,并将其写入文本文件
Feature feature;
while ((feature = layer.GetNextFeature()) != null)
{
string geometry = feature.GetGeometryRef().ExportToWkt();
file.WriteLine(geometry);
feature.Dispose();
}
// 关闭文件和数据源
file.Close();
ds.Dispose();
```
这段代码假设你已经安装了 GDAL 库并将其添加到了项目中。如果你还没有安装,可以在 GDAL 官网下载并安装。
相关问题
c#将shp文件保存为txt文件
你可以使用DotSpatial库来读取和处理shp文件,然后使用C#的文件写入方法将数据保存为txt文件。
以下是一个示例代码:
```csharp
using DotSpatial.Data;
// 读取shp文件
FeatureSet featureSet = FeatureSet.Open("path/to/your/shp/file.shp");
// 获取属性表的列名
List<string> columnNames = featureSet.DataTable.Columns.Cast<DataColumn>()
.Select(x => x.ColumnName).ToList();
// 打开txt文件进行写入
using (StreamWriter writer = new StreamWriter("path/to/your/txt/file.txt"))
{
// 写入列名
writer.WriteLine(string.Join(",", columnNames));
// 写入每个要素的属性值
foreach (IFeature feature in featureSet.Features)
{
string[] values = feature.DataRow.ItemArray
.Select(x => x.ToString()).ToArray();
writer.WriteLine(string.Join(",", values));
}
}
```
这段代码将会把shp文件中的属性表数据保存为一个逗号分隔的txt文件,其中第一行为列名,每个后续行代表一个要素的属性值。
用c#将shp文件保存为txt格式文件
您好!以下是使用C#将shp文件保存为txt格式文件的示例代码:
```csharp
using OSGeo.OGR;
public void SaveShpToTxt(string shpFilePath, string txtFilePath)
{
// 注册OGR驱动
Ogr.RegisterAll();
// 打开shp文件
DataSource ds = Ogr.Open(shpFilePath, 0);
Layer layer = ds.GetLayerByIndex(0);
// 创建txt文件
StreamWriter sw = new StreamWriter(txtFilePath);
// 逐个读取feature并保存到txt文件中
Feature feature = null;
while ((feature = layer.GetNextFeature()) != null)
{
Geometry geometry = feature.GetGeometryRef();
if (geometry.GetGeometryType() == wkbGeometryType.wkbPoint)
{
string x = geometry.GetX(0).ToString();
string y = geometry.GetY(0).ToString();
sw.WriteLine(x + "," + y);
}
}
// 关闭txt文件
sw.Close();
}
```
其中,`shpFilePath`是要转换的shp文件路径,`txtFilePath`是要保存的txt文件路径。上述代码只是示例,您可以根据自己需要修改代码,例如修改保存的要素类型等。
需要注意的是,在使用OGR库时需要引入`OSGeo.OGR`命名空间,并且需要将OGR的C++库文件复制到程序运行的目录中。
希望这能帮到您!
阅读全文
相关推荐


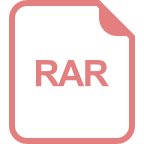
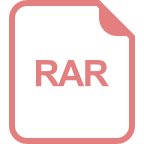
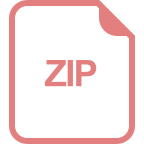
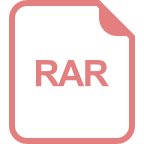
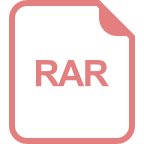
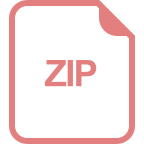


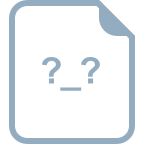
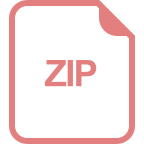