帮我写一段c++ opencv的匹配图像拼接代码
时间: 2023-10-20 08:15:09 浏览: 44
以下是一个简单的OpenCV图像拼接代码,它将两个图像水平拼接在一起:
```c
#include <opencv2/opencv.hpp>
#include <iostream>
int main(int argc, char** argv)
{
// 读取两个图像
cv::Mat image1 = cv::imread("image1.jpg");
cv::Mat image2 = cv::imread("image2.jpg");
// 图像拼接
cv::Mat result;
cv::hconcat(image1, image2, result);
// 显示结果
cv::imshow("Result", result);
cv::waitKey(0);
return 0;
}
```
在上面的代码中,`cv::imread("image1.jpg")`和`cv::imread("image2.jpg")`分别读取两个图像。然后,使用`cv::hconcat`函数将两个图像水平拼接在一起,并将结果存储在`result`变量中。最后,使用`cv::imshow`函数显示结果,并使用`cv::waitKey`等待用户按下任意键关闭窗口。
请注意,这只是一个简单的示例代码,可能需要根据您的应用程序进行修改。例如,您可能需要对图像进行预处理或更复杂的拼接操作。
相关问题
帮我写一段c++ opencv的图像拼接代码
以下是一段简单的 OpenCV 图像拼接代码,将两张图像水平方向拼接在一起:
```
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat img1 = imread("image1.jpg");
Mat img2 = imread("image2.jpg");
// 获取图像宽度和高度
int width = img1.cols + img2.cols;
int height = max(img1.rows, img2.rows);
// 创建新图像
Mat new_img(height, width, CV_8UC3);
// 将两张图像拷贝到新图像中
img1.copyTo(new_img(Rect(0, 0, img1.cols, img1.rows)));
img2.copyTo(new_img(Rect(img1.cols, 0, img2.cols, img2.rows)));
// 显示拼接后的图像
imshow("Image Stitching", new_img);
waitKey(0);
return 0;
}
```
上述代码中,首先使用 `imread` 函数读取两张图像,并计算它们的宽度和高度。然后,通过创建一个新的图像 `new_img`,使用 `copyTo` 函数将两张图像拷贝到新图像的指定位置上,最后将拼接后的图像显示出来。
帮我写一段c++ opencv的匹配图像拼接融合代码
以下是一个简单的基于OpenCV的图像拼接融合代码:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main(int argc, char** argv)
{
// 读取需要拼接的图像
Mat img1 = imread("image1.jpg");
Mat img2 = imread("image2.jpg");
// 将两张图像拼接在一起
Mat stitchedImage;
Ptr<Stitcher> stitcher = Stitcher::create();
stitcher->stitch(vector<Mat>{img1, img2}, stitchedImage);
// 显示拼接后的图像
namedWindow("Stitched Image", WINDOW_NORMAL);
imshow("Stitched Image", stitchedImage);
waitKey(0);
return 0;
}
```
这个代码使用了OpenCV自带的`Stitcher`类来实现图像拼接。首先,我们读取需要拼接的两张图像。然后,我们创建了一个`Stitcher`对象,并调用`stitch`函数将两张图像拼接在一起。最后,我们显示了拼接后的图像。
需要注意的是,这个简单的代码只能处理两张图像的拼接。如果你需要拼接更多的图像,你需要使用`Stitcher`类的其他函数,并对图像进行预处理和校正操作,以获得更好的拼接效果。
相关推荐
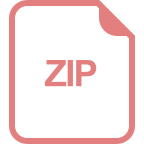
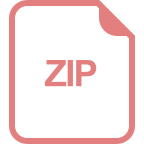
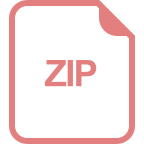
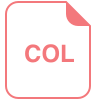
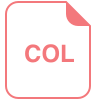
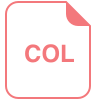
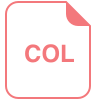
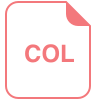







